Welcome back to Digital Academy, the Complete Python Development Course for Beginners.
In the previous tutorial, You may have discovered the Basic Data Types in Python, including: Numbers, and Strings. As well as some advanced Collections, which are: Lists, Dictionaries, Tuples, and Sets. Most important: how to use them, and what are some of their advantages. In this entire new video, you will now discover: Syntax and Variables, in Python.
You might have produced a “Hello, World!” program, in your chosen programming language. It is all very well, but quite limited, right?!
More complex programs need a way of labelling data with a name, so it can be referenced later. This has the rather wonderful side-effect, of making computer code easier to read!
Nonetheless, there are a certain rules and regulations we have to follow, while writing a variable. Let’s take a look at the variable definition, to understand how we declare a variable, in Python.
Variable Definition

In a programming language, a variable is a memory location. Think of a variable as a name, attached to a particular object. Variables are like containers, for storing data values. The value that you have stored into this container may change in the future, according to the specifications.
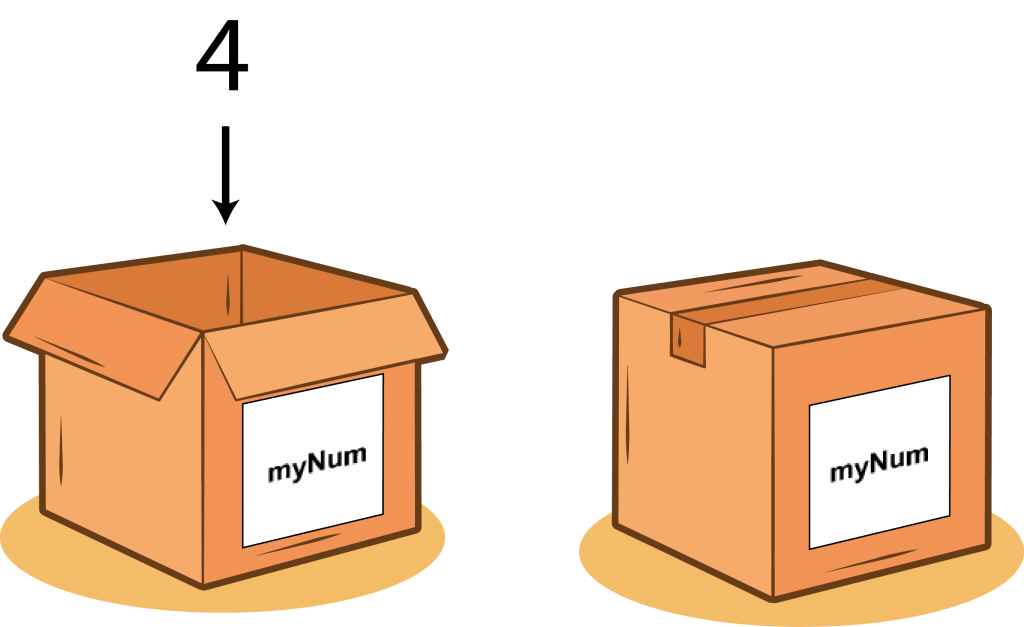
Variable Declaration
In Python, variables do not need to be defined or declared in advance, with any particular type – as is the case, in many other programming languages. Variables can even change type, after they have been set. A variable is created, as soon as a value is assigned to it, and then you can start using it. Assignment, is done with a single equals sign.
>>> n = 300
This is read or interpreted as “n is assigned the value 300.” Once this is done, n can be used in a statement or expression, and its value will be substituted.
>>> print(n)
300
Later, if you change the value of n and use it again, the new value will be substituted, instead.
>>> n = 1000
>>> print(n)
1000
>>> n
1000
Python also allows chained assignment, which makes it possible to assign the same value, to multiple variables. The chained assignment above, assigns 300 to the variables a, b, and c, simultaneously.
>>> a = b = c = 300
>>> print(a, b, c)
300 300 300
Object References
What is actually happening, when you make a variable assignment? This is an important question in Python, because the answer differs somewhat from what you would find, in many other programming languages!
Python is a highly object-oriented language. In fact, virtually every item of data in a Python program is an object, of a specific type, or class. This point will be reiterated many times, over the course of these tutorials.
>>> print(300)
300
Consider this code. When presented with the statement print(300), the interpreter does the following steps:
- Creates an integer object
- Gives it the value 300
- Displays it to the console
You can see that an integer object is created, using the built-in type() function.
>>> type(300)
<class 'int'>
Variable Assignment
A Python variable is a symbolic name, that is a reference or pointer to an object. Once an object is assigned to a variable, you can refer to the object, using that name. But the data itself is still contained, within the object.
>>> n = 300
For example. This assignment creates an integer object, with the value 300, and assigns the variable n to point to that object.
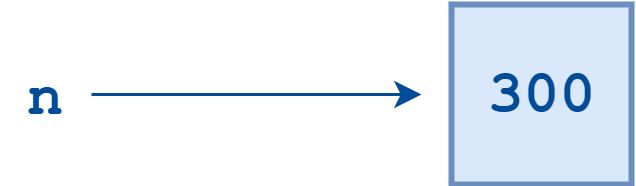
The following code verifies that n points to an integer object:
>>> print(n)
300
>>> type(n)
<class 'int'>
Multiple References to a Single Object
Now consider the following statement:
>>> m = n
What happens, when it is executed? Python does not create another object. It simply creates a new symbolic name or reference, m, which points to the same object, that n points to.

References to Separate Objects
Next, suppose you do this:
>>> m = 400
Now Python creates a new integer object with the value 400, and m becomes a reference to it.

Orphaned Object
Lastly, suppose this statement is executed next:
>>> n = "foo"
Now Python creates a string object with the value “foo” and makes n reference that.
There is no longer any reference to the integer object 300. It is now orphaned, and there is no way to access it.

Tutorials in this series will occasionally refer to the lifetime, of an object. An object’s life, begins when it is created. At which time, at least one reference to it is created. During an object’s lifetime, additional references to it may be created, and references to it may be deleted, as well. An object stays alive, as long as it has at least one reference to it.
When the number of references to an object drops to zero, it is no longer accessible. At that point, its lifetime is over. Python will eventually notice that it is inaccessible and reclaim the allocated memory, so it can be used for something else. In computer lingo, this process is referred to as “garbage collection”.
Object Identity
In Python, every object that is created is given a number that uniquely identifies it. It is guaranteed that no two objects will have the same identifier, during any period in which their lifetimes overlap. Once an object’s reference count drops to zero and it is garbage collected, then its identifying number becomes available – and may be used again.
The built-in Python function id() returns an object’s identifier. Using this function, you can verify that two variables point to the same object, indeed!
After the assignment m = n, m and n both point to the same object, confirmed by the fact that id(m) and id(n) return the same number. Once m is reassigned to 400, m and n point to different objects, with different identities!
>>> n = 300
>>> m = n
>>> id(n)
60127840
>>> id(m)
60127840
>>> m = 400
>>> id(m)
60127872
Variable Names & Syntax
The examples you have seen so far, have used short variable names. But the truth is, that variable names can be more descriptive. In fact, it is usually beneficial if they are, because it makes the purpose of the variable more evident, at first glance! At least you can tell from the name, what the value of the variable is supposed to represent.
Officially, variable names in Python can be any length, and can consist of uppercase and lowercase letters, digits, and the underscore character. An additional restriction is that, although variable name can contain digits, the first character of a variable name cannot be one. Moreover, variables names are case-sensitive, which means that age, Age and AGE are three different variables.
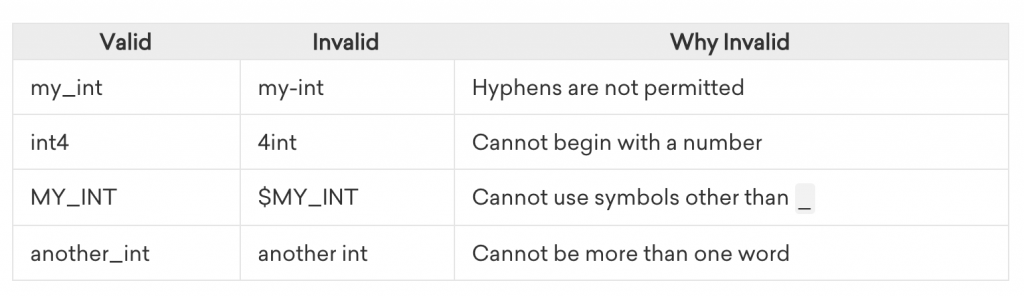
For example, which of the following variable names are valid? You may have guessed right. If not, just playback and refer to the previous 5 rules, or even The Style Guide for Python Code, also known as PEP 8, which contains Naming Conventions that list suggested standards for names, of different object types!
There is nothing stopping you from creating multiple different variables in the same program, with the same name. But it is probably ill-advised. It would certainly be likely to confuse anyone trying to read your code, and even you yourself, after you had been away from it awhile!
Thus, It is worthwhile, to give a variable a name that is descriptive enough, to make clear what it is being used for. You will see later, that variables are not the only things, that can be given names. Actually, you can also name: functions, classes, modules, and so on. The rules that apply to variable names, also apply to identifiers.
Reserved Keywords
There is one more restriction, on identifier names. The Python language reserves a small set of keywords, that designate special language functionality. No object can have the same name, as a reserved word.
In Python 3, there are currently 33 reserved keywords, as described in this table. You can see this list any time, by typing help(“keywords”) to the Python interpreter. Reserved words are case-sensitive, and must be used exactly as shown. They are all entirely lowercase, except for: False, None, and True. Trying to create a variable with the same name as any reserved word, results in a error: SyntaxError.

Variable Types
In many programming languages, variables are statically typed. That means a variable is initially declared to have a specific data type, and any value assigned to it during its lifetime, must always have that type!
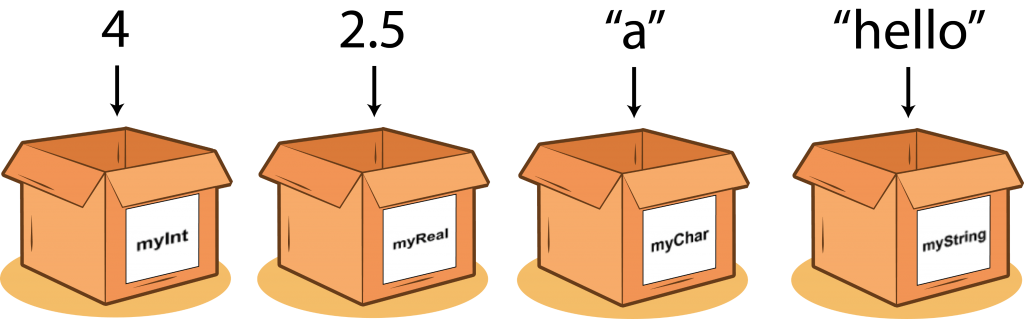
Variables in Python are not subject to this restriction. In Python, a variable may be assigned a value of one type, and then later re-assigned a value, of a different type. If you are interested in learning Basic and Advanced Data Types in Python: Please, check out this suggested video!
Being able to reassign a value into a variable, can be very useful. Throughout the course of a program, you may need to accept user-generated values into already initialised variables, or may have to change the assignment to something you previously defined!
>>> var = 23.5
>>> print(var)
23.5
>>> var = "Now I'm a string"
>>> print(var)
Now I'm a string
Variable Scope
If you are familiar with Python, or any other programming languages, you will certainly know that: variables need to be defined, before they can be used in your program. Depending on how and where it was defined, a variable will have to be accessed, in different ways.
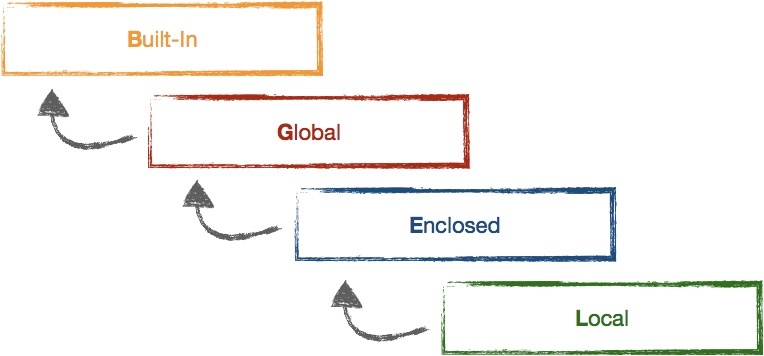
Not all variables are accessible, from all parts of your program. And not all variables exist, for the same amount of time! Where a variable is accessible, and how long it exists, depend on how it is defined. We call the part of a program where a variable is accessible, its scope. And the duration for which the variable exists, its lifetime.
The scope of a variable, refers to the places where you can see or access a variable. Some variables are defined globally, others locally. This means that a variable referring to an entity in a certain part of a program, may refer to something different, in another part of the program, even at different points in time!
Global Variables
Thus, if you define a variable at the top of your script, it will be a global variable. This means that it is accessible, from anywhere in your script: including from a class, defined in the same module. Or both, inside and outside of a function.

Local Variables
On the other hand, A variable which is defined inside a function, is local to that function. It is accessible from the point at which it was defined, until the end of the function. And it exists for as long as the function is executing. For instance, the parameter names in the function definition, behave like local variables. But they contain the values that we pass into the function, when we call it. When you use the assignment operator equals inside a function, its default behaviour is to create a new local variable, unless a variable with the same name is already defined, in the local scope.

Normally, when you create a variable inside a function, that variable is local, and can only be used inside that function. If you create a variable, with the same name inside a function, the global variable with the same name, will remain as it was – and will keep its original value. Thus, in order to create a global variable inside a function, you can use the global reserved keyword. Also, use the global keyword if you want to change: a global variable inside a function, but that was declared outside.
Conclusion
This tutorial covered the Basics of Python variables, including object references and identity, as well as naming of Python identifiers. You should now have a good understanding of some of Python’s Data Types, and know How to create variables, that reference objects of those Data Types.
In the next video, you will discover and may learn how to combine data objects into expressions, involving various conditions and operators. So, Do not waste any minute, and join us in the next episode!
– Digital Academy™ 🎓
📖Complete Python Development Course for Beginners
#Python #Tutorial #Beginners #Syntax #Variables
***
♡ Thanks for watching and supporting ♡
Please Subscribe. Hit the notification bell.
Like, Comment and Share.
***
♡ FOLLOW US ♡
✧ http://digital.academy.free.fr/
✧ https://twitter.com/DigitalAcademyy
✧ https://www.facebook.com/digitalacademyfr
✧ https://www.instagram.com/digital_academy_fr
✧ https://www.youtube.com/c/DigitalAcademyOnline
***
♡ SUPPORT US ♡
✧ http://digital.academy.free.fr/join
✧ http://digital.academy.free.fr/donate
✧ http://digital.academy.free.fr/subscribe
✧ https://www.patreon.com/digital_academy
✧ https://www.buymeacoffee.com/digital_academy