Welcome back to Digital Academy, the Complete Python Development Course for Beginners.
In the previous tutorial, you may have discovered how to install and configure Python, on your PC or Mac. But also the few steps to download your new favourite Code Editor, or your Integrated Development Environment, so you can start practicing straight away. In this new video, you will discover the Variables and Data Types in Python. So, Let’s play this video!
Data Types (Numbers, String, Tuple, List, Dictionary, Set)
Now that you know how to interact with the Python interpreter, and execute Python code, it is time to dig into the basic Data Types, in Python. Data Types, are the classification or categorization of Data Items. It represents the kind of value that tells what operations can be performed, on a particular data.
Thus: Every value in Python has a Data Type. Since everything is an object in Python, Data Types are actually Classes, and Variables are Instances (Object) of these classes. Consequently, There are multiple Data Types in Python. Some of the most basic Data Types built into Python are: Numeric, String, and Boolean types. Others are part of the Collections: Tuples, Lists, Dictionaries, and Sets.

Mutable / Immutable Objects
When choosing a Data or Collection Type, it is useful to understand the properties of that type. Choosing the right type, for a particular Data Set, could mean an increase in efficiency, or in security. In fact: Objects of these types are stored in a computer’s memory, for processing. Some of these values can be modified during processing, but contents of others cannot be altered, once they are created in the memory.
- Number values, Strings, and Tuple are immutable, which means their contents cannot be altered after creation!
- On the other hand, collection of items in a List or Dictionary object can be modified. It is possible to add, delete, insert, and even rearrange items. Hence, they are mutable objects.
Are you wondering: What Data Type you should use? Don’t worry, By the end of this tutorial, you will be familiar with: What objects of these types look like, and How to represent them.
Numerical Data Types
In Python, numeric data type represent the data which has numeric value. Numeric value can be: Integer or Floating numbers, even Complex numbers. These values are defined as int, float and complex class in Python. We can use the type() function, to know which class a variable or a value belongs to. And the isinstance() function, to check if an object belongs to a particular class. You can convert from one type to another with the int(), float(), and complex() methods.
Integers
This value is represented by int class. It contains positive or negative whole numbers (without fraction or decimal). In Python 3, Integers can be of any length, it is only limited by the amount of memory your system has, as are all things, but beyond that, an integer can be as long as you need it to be:
>>> print(123123123123123123123123123123123123123123123123 + 1)
123123123123123123123123123123123123123123123124
Python interprets a sequence of decimal digits without any prefix to be a decimal number. But the following strings can be prepended to an integer value, to indicate a base other than 10:
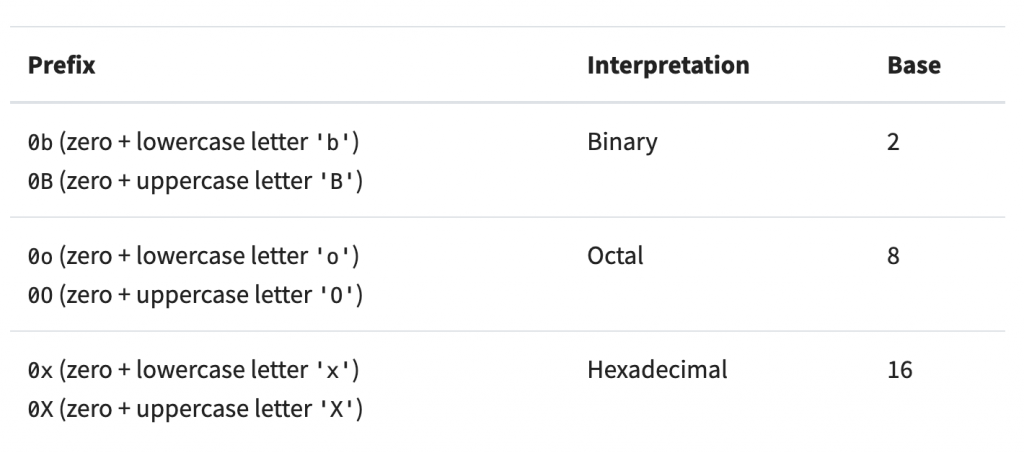
For example:
>>> print(10)
10
>>> print(0o10)
8
>>> print(0x10)
16
>>> print(0b10)
2
The underlying type of a Python integer, irrespective of the base used to specify it, is called int:
>>> type(10)
<class 'int'>
>>> type(0o10)
<class 'int'>
>>> type(0x10)
<class 'int'>
Note: This is a good time to mention that if you want to display a value while in a Python Shell, you don’t need to use the print() function. Just typing the value at the 3 greater-than signs prompt, and hitting Enter, will display it:
>>> 10
10
>>> 0x10
16
>>> 0b10
2
Note that this does not work inside a script file. A value appearing on a line by itself in a script file will not do anything.
Floating-Point Numbers
This value, represented by float class, designates a floating-point number. It is a real number, with the floating point representation, and is specified with a decimal point. Optionally, the character ‘e’, followed by a positive or negative integer, may be appended, to specify the scientific notation. This representation is accurate up to 15 decimal places.
>>> 4.2
4.2
>>> type(4.2)
<class 'float'>
>>> 4.
4.0
>>> .2
0.2
>>> .4e7
4000000.0
>>> type(.4e7)
<class 'float'>
>>> 4.2e-4
0.00042
Complex Numbers
Complex Numbers, represented by complex class, is written in the form, (real part) + (imaginary part)j. It is stored internally, using either rectangular or Cartesian coordinates. It can be very useful if you are studying or working with mathematical functions, or in engineering. For example: 2+3j
>>> 2+3j
(2+3j)
>>> type(2+3j)
<class 'complex'>
Boolean
Python 3 provides a Boolean data type. Objects of Boolean type may have one of two values, True or False
In programming you often need to know if an expression is True or False. You can evaluate any expression in Python, and get one of these two answers. For instance, when you compare 2 values, the expression is evaluated, then Python returns the Boolean answer. The same behaviour occurs, when you run a condition in an if statement.
Python also has many built-in functions that returns a boolean value, like the isinstance() function, which can be used to determine if an object is of a certain data type. You will learn more about evaluation of objects in the Boolean context, when you encounter logical operators, in the upcoming tutorial on operators, and expressions in Python.
>>> type(True)
<class 'bool'>
>>> type(False)
<class 'bool'>
Strings
The string type in Python, is represented by strclass. Strings are immutable, which means that their content cannot be altered, after their creation.
Like many other popular programming languages, strings in Python are arrays of bytes – which represents unicode characters. However, Python does not have a character data type, a single character is also considered as a string – with a length of 1!
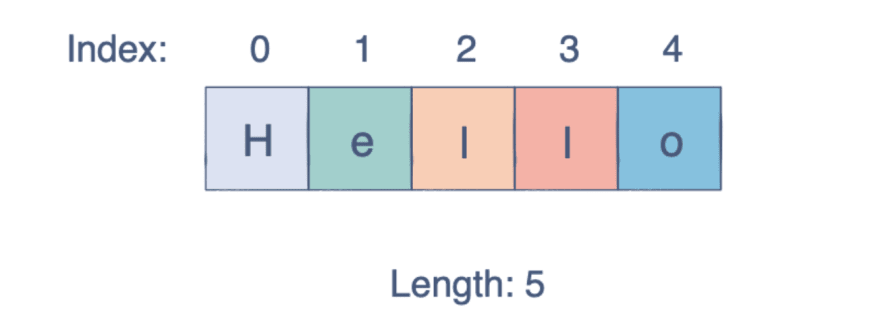
We denote or declare the string values inside single quotes, or double quotes – ‘hello’ is the same as “hello”. Multi-line strings can be denoted using triple quotes.
>>> print("I am a string.")
I am a string.
>>> type("I am a string.")
<class 'str'>
>>> print('I am too.')
I am too.
>>> type('I am too.')
<class 'str'>
Like list and tuple, slicing operator can be used with strings. To access the values in a string, we use the indexes and square brackets. Python also has a set of built-in methods that you can use on strings: strip(), lower(), upper(), replace(), split(), among so many others.
Lists
Now that you have understood numbers and strings, Let’s go through the relatively more complex data types.
A list is one of the most used datatype in Python, and is very flexible. It is a collection of items, which is ordered, and mutable. Which means that values of a list can be altered, even after its creation. All the elements in a list are indexed according to a definite sequence. The indexing of a list is done with 0 being the first index. Each element in the list has its own place, which allows duplicating of elements.
Lists in Python are just like the arrays, declared in other languages. All the items in a list do not need to be of the same type, which makes one of the most powerful tool in Python.
Declaring a list is pretty straight forward. Items, separated by commas, are enclosed within square brackets. A single list may contain Data Types like: Integers, Strings, as well as Objects. Lists in Python are ordered, and have a definite count. You can use the slicing operator to access and extract an item from a list, by referring to the index number.
>>> d = [1, 2, "Three", Object]
>>> type(d)
<class 'list'>
Dictionaries
Dictionary in Python is an unordered and indexed collection of values, used to store data. Unlike other Data Types, that hold only single value as an element, dictionary holds key:value pair. Key-value is provided in the dictionary to make it more optimized for retrieving data. It is generally used when you have a huge amount of data, since you must know the key, to retrieve the value – but not the other way around!
In Python, a Dictionary can be created by placing a sequence of elements within curly braces. Dictionary can also be created by the built-in function, dict(). Values in a dictionary can be of any data type, and can be duplicated, whereas keys cannot be repeated. Each key-value pair is separated by a colon, whereas each entry is separated by a comma.
>>> d = {1: 'value', 'key': 2}
>>> type(d)
<class 'dict'>
Note – Dictionary keys are case sensitive. Same name but different cases of Key will be treated distinctly.
Tuples
Tuple is an ordered collection of Python objects, much like a List. The sequence of values stored in a tuple can be of any type, and they are indexed by integers, which allows duplicate members. The important difference between a list and a tuple, is that tuples are immutable. Tuples, once created, cannot be modified. Tuples are used to write-protect data, and are usually faster than list – as it cannot change dynamically.
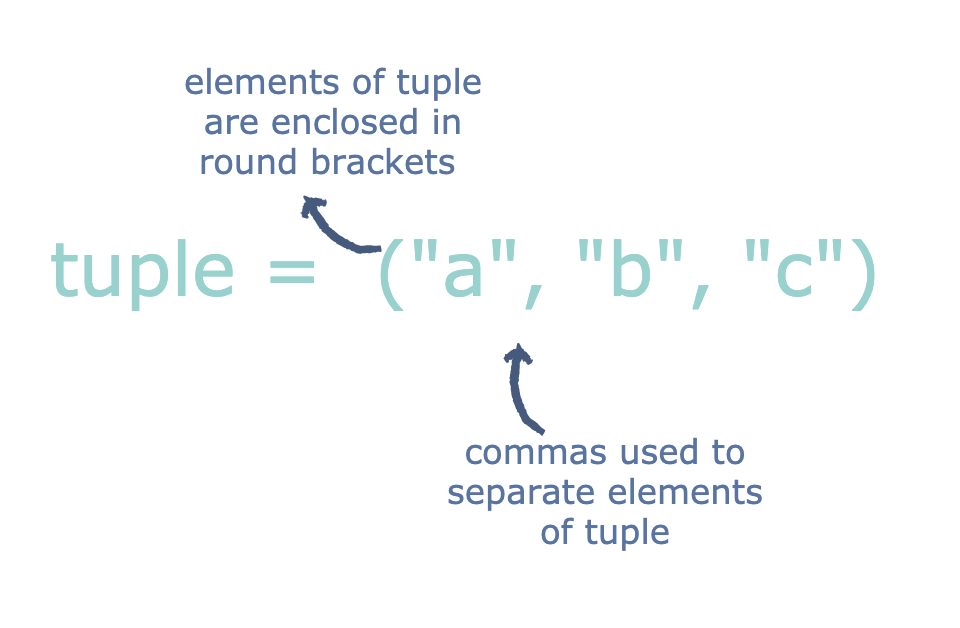
In Python, tuples are represented by tuple class, and created by placing sequence of values separated by comma, with or without the use of parentheses for grouping of data. Tuples can contain any number of elements, and of any datatype, like: strings, integers, list, etc. You can use the slicing operator to extract items, but cannot change values
>>> d = (1, 2, "Three", Object)
>>> type(d)
<class 'tuple'>
Sets
In Python, Set is an unordered collection of data type that is: iterable, mutable and has unique elements. The order of elements in a set is undefined, though it may consist of various elements. The advantage of using a set, as opposed to a list, is that it has a highly optimized method, for checking whether a specific element is contained in the set!
Set is defined by curly braces, with unique values, which are separated by commas. You can perform multiple set operations, like: union and intersection on two sets. Since sets are unordered collection, indexing has no meaning. Hence the slicing operator does not work.
>>> s = {1, 2, 2, 3, 3, 3}
s{1, 2, 3}
>>> type(s)
<class 'set'>
Conversion between Data Types
In Python, Data Types are used to classify one particular type of data, determining the values that you can assign to the type, and the operations you can perform on it. Nonetheless, there are few times you need to convert values, between types, in order to manipulate values in a different way. For example, you may need to concatenate numeric values with strings, or represent decimal places in numbers that were initialized as integer values.
As you have seen along the previous examples, you can get the data type of any object, by using the type() function. And the isinstance() function, to check whether an object belongs to a particular class.
You also have multiple constructors, for each one of the data types in Python. You can simply use these constructors, to use the specified data type. Or, you can change a data type to another one, using these constructors. Basically, type casting is the process of changing one data type, into another.
Using these constructors, you can use various data types, with the functionality of the other. Suppose you declare the list mentioned in the example, as a tuple in a program. It will become immutable for that particular operation. Similarly, we can use other constructors, as well!
- list()
- set()
- tuple()
- dict()
- str()
- int()
- float()
- bool()
- complex()
Conclusion
In this tutorial, you have learned about the built-in Data Types, that Python provides. The examples given so far have all manipulated and displayed only constant values. In most programs, you are usually going to want to create objects that change in value, as the program executes. Head to the next tutorial, and learn about Python variables.

– Digital Academy™ 🎓
📖Complete Python Development Course for Beginners
#Python #Tutorial #Beginners #Variables #DataTypes
***
♡ Thanks for watching and supporting ♡
Please Subscribe. Hit the notification bell.
Like, Comment and Share.
***
♡ FOLLOW US ♡
✧ http://digital.academy.free.fr/
✧ https://twitter.com/DigitalAcademyy
✧ https://www.facebook.com/digitalacademyfr
✧ https://www.instagram.com/digital_academy_fr
✧ https://www.youtube.com/c/DigitalAcademyOnline
***
♡ SUPPORT US ♡
✧ http://digital.academy.free.fr/join
✧ http://digital.academy.free.fr/donate
✧ http://digital.academy.free.fr/subscribe
✧ https://www.patreon.com/digital_academy
✧ https://www.buymeacoffee.com/digital_academy