Welcome back to Digital Academy, the Complete Python Development Course for Beginners.
In the previous tutorial, you may have discovered Python Iterations: FOR and WHILE Loops, which are one of the core concepts, when you want to repeat a block of code multiple times – either for a fixed number of times, or indefinitely. In this brand new video, you will now discover Dictionary in Python, so you can: create it, add and remove an item, iterate through a dictionary, seeking for and accessing an indexed item, inside of the collection – plus various built-in methods.
Please, Do not forget to smash that Like button, for the YouTube algorithm – since it does really help supporting us, and providing new FREE content, once a week! You may also want to follow Digital Academy, on our other social platforms: Facebook, Instagram, Twitter and Patreon. This way, we could: stay in touch, grow this community, then share and help each other, throughout this exciting journey, OUR journey! Are you interested to be part of this community? All the Links are in the description below! Now, Let’s play this video …
Definition
Dictionary in Python is a collection of items, which is unordered, indexed by keys and mutable – so it can be changed, once it has been created. While other Data Types have value as an element, a dictionary has a “key: value” pair, where each key is mapped to a single value. Consequently, dictionaries are optimised to get values, when the key is known!
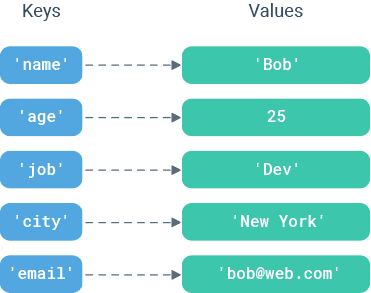
The important properties of Python dictionaries, are as follows:
- Dictionaries are unordered – Items stored in a dictionary are not kept in any particular order.
- Dictionaries are mutable – They can be changed in place, can grow and shrink – on demand.
- Accessed by keys – Dictionary items are accessed by keys (indexed), not by their position (index).
- Dictionaries can be nested – A dictionary can contain another dictionary – and so forth (Nested Dictionary).
Great! Now that you have understood what a dictionary is, let’s move forward and discover how to create a dictionary in Python, but also what are all of the operations you can perform on it, in order to: add, remove, or access an item, or even search for a specific item inside of the dictionary.
Creating
Creating a dictionary in Python is as simple as placing “key: value” items inside curly braces {}, separated by comma. Each key is separated from its associated value, by a colon “:”. While values can be of any data type and can repeat, keys must be unique, and of immutable type – like string, number or tuple with immutable elements.
Let’s have a closer look at its syntax, so you can declare your own dictionary. Here is an example, in which you want to store an employee record. First, Let’s declare an empty dictionary – using curly braces {}. Eventually, you will add all of the personal information about the employee, so you can store its information and then access it, later.
employee = {
'age': 27,
'city': 'Paris',
'job': 'Youtuber',
'name': 'Digital Academy',
'email': 'digital.academy@free.fr'
}
You can also convert two-value sequences into a dictionary, using the built-in function dict(). The first item inside each sequence is then used as the key, and the second as the value.
# Using dict() constructor
my_dict = dict({'name':'apple', 2:'strawberry'})
# From sequence, having each item as a pair
my_dict = dict([('name','Digital Academy'), ('age',27)]) # List of Tuples
my_dict = dict((['name','Digital Academy'], ['age',27])) # Tuple of Lists
# my_dict = {'name': 'Digital Academy', 'age': 27}
When the keys are simple strings, it is sometimes easier to specify key:value pairs, using keyword arguments.
my_dict = dict(
age = 27,
job = 'Youtuber',
name = 'Digital Academy'
)
# my_dict = {'name': 'Digital Academy', 'age': 27, 'job': 'Youtuber'}
Dictionaries are pretty straightforward, but here are a few points you should be aware of, when using them.
Keys must be unique
A key can appear in a dictionary only once. Even if you specify a key more than once during the creation of a dictionary, the last value for that key becomes the associated value. Notice that the 1st occurrence of the key ‘name’ (Jérémy) has been replaced by the second one (Digital Academy).
my_dict = {
'age': 27,
'name': 'Jérémy',
'name': 'Digital Academy'
}
# my_dict = {'name': 'Digital Academy', 'age': 27}
Key must be immutable type
You can use any object of immutable type as dictionary keys – such as numbers, strings, booleans, tuples. Otherwise, an exception is raised – when mutable object is used as a key.
my_dict = {
0: 1, # Number
True: 'a', # Boolean
(2,2): 25, # Tuple
'name': 'Digital Academy' # String
}
my_dict = {
0: 1, # Number
True: 'a', # Boolean
[2,2]: 25, # TypeError: unhashable type: 'list'
'name': 'Digital Academy' # String
}
Value can be of any type
There are no restrictions on dictionary values. A dictionary value can be any type of object, and can even appear in a dictionary, multiple times.
# Values of different datatypes
my_dict = {
'a': [1,2,3], # List
'b': {1,2,3} # Set
}
# Duplicate values
my_dict = {
'a': [1,2], # List a
'b': [1,2], # List b
'c': [1,2] # List c
}
Other Ways to Create Dictionaries
There are lots of other ways to create a dictionary. You can use dict() function along with the zip() function, to combine separate lists of keys and values obtained dynamically, at runtime.
Example: Create a dictionary with list of zipped keys/values
keys = ['name', 'age', 'job']
values = ['Digital Academy', 27, 'Youtuber']
my_dict = dict(zip(keys, values))
# my_dict = {'name': 'Digital Academy', 'age': 27, 'job': 'Youtuber'}
You will often want to create a dictionary with default values for each key. The fromkeys() method offers a way to do it.
Example: Initialize dictionary with default value ‘0’ for each key
keys = ['a', 'b', 'c']
defaultValue = 0
my_dict = dict.fromkeys(keys, defaultValue)
# my_dict = {'a': 0, 'b': 0, 'c': 0}
Adding
Adding or Updating dictionary’s items is very easy. Dictionaries are mutable, which means you can add new items or change the value of an existing item using assignment operator. If the key is already present, the value gets updated – else, a new “key: value” pair is added into the dictionary.
Adding an entry to an existing dictionary is simply a matter of assigning a new key and value pair. If the key is new, it is added to the dictionary with its value. Example: Add new item ‘city’ to the dictionary
my_dict = {
'age': 27,
'job': 'Youtuber',
'name': 'Digital Academy'
}
my_dict['city'] = 'Paris'
# my_dict = {'age': 27, 'job': 'Youtuber', 'city': 'Paris', 'name': 'Digital Academy'}
If the key is already present in the dictionary, its value will be replaced by the new one. Thus, if you want to update an entry, you can just assign a new value to an existing key. Example: Change the value of the key ‘name’
my_dict = {
'age': 27,
'city': 'Paris',
'job': 'Youtuber',
'name': 'Digital Academy'
}
my_dict['name'] = 'Jérémy'
# my_dict = {'age': 27, 'city': 'Paris', job': 'Youtuber', 'name': 'Jérémy'}
Accessing
Of course, dictionary elements must be accessible, somehow! If you do not get them by index, then how would you get them? While indexing is used with other container types to access values, dictionary use keys. Values can be retrieved from a dictionary by specifying its corresponding key – in square brackets []. Example: Print the value of the key ‘name’
my_dict = {
'age': 27,
'job': 'Youtuber',
'name': 'Digital Academy'
}
print(my_dict['name'])
# OUTPUT: Digital Academy
Nonetheless, If you refer to a key that is not in the dictionary, you will get an exception. To avoid such exception, you can use the special dictionary get() method. The difference while using get() is that it returns None, instead of KeyError, if the key is not found – and returns the value associated to its key, if key is in the dictionary.
Example: Try to print the value of the key ‘salary’
print(my_dict['salary'])
# OUTPUT: KeyError: 'salary'
Example: Print values of the keys ‘name’ and ‘salary’
# Key present
print(my_dict.get('name')) # OUTPUT: Digital Academy
# Key absent
print(my_dict.get('salary')) # OUTPUT: None
Removing
There are several ways to remove items from a dictionary: either by its key, the last inserted item, a random item, or even remove all of them – at once.
Remove an Item by Key
If you know the key of the item you want: you can remove a particular item in a dictionary, by using the function pop(). This function removes an item with the provided key, and returns its value.
my_dict = {
'age': 27,
'job': 'Youtuber',
'name': 'Digital Academy'
}
x = my_dict.pop('age')
# x = 27
# my_dict = {'job': 'Youtuber', 'name': 'Digital Academy'}
If you do not need to keep nor use the removed value, you can also use the del keyword to remove individual items. To delete an entry, use the del statement, specifying the key to delete.
my_dict = {
'age': 27,
'job': 'Youtuber',
'name': 'Digital Academy'
}
del my_dict['age']
# my_dict = {'job': 'Youtuber', 'name': 'Digital Academy'}
Remove Last Inserted Item
Sometimes you need to iterate through a dictionary in Python and delete its items sequentially. To accomplish this task, you can use the function popitem(), which will remove and return the last inserted item from the dictionary. Please note that in Python versions before 3.7, the popitem() method removes a random item – not necessarily the last inserted!
my_dict = {
'age': 27,
'job': 'Youtuber',
'name': 'Digital Academy'
}
name = my_dict.popitem()
print(my_dict) # {'age': 27, 'job': 'Youtuber'}
# Removed pair
print(name) # ('name', 'Digital Academy')
Remove all Items
All the items can be removed at once, using the clear() method. Consequently, it will delete all keys and values from the dictionary. You can also use the del keyword, to remove the entire dictionary itself.
my_dict = {
'age': 27,
'job': 'Youtuber',
'name': 'Digital Academy'
}
my_dict.clear()
# my_dict = {}
Listing Keys / Values
There are three dictionary methods that return all of the dictionary’s keys, values and key-value pairs: keys(), values(), and items(). These methods are useful in loops that need to step through dictionary entries, one by one. All the three methods return iterable object.
- keys(): Returns an iterable object containing the list of all the keys inside the dictionary.
- values(): Returns an iterable object containing the list of all the values inside the dictionary.
- items(): Returns a list of tuples, with all “key: value” pairs – [(key1, value1), (key2, value2), …]
Note: If you want a true list from these methods, you can wrap them in a list() function.
my_dict = {
'age': 27,
'job': 'Youtuber',
'name': 'Digital Academy'
}
# Get all Keys
print(list(my_dict.keys()))
# OUTPUT = ['age', 'job', 'name']
# Get all Values
print(list(my_dict.values()))
# OUTPUT = [27, 'Youtuber', 'Digital Academy']
# Get all Items (key, value)
print(list(my_dict.items()))
# OUTPUT = [('age', 27), ('job', 'Youtuber'), ('name', 'Digital Academy')]
Checking existence
If you want to know if a key exists in a dictionary, use the “in” and “not in” operators – with if statement. You can also use keys() method, to check whether your key is part of the dictionary’s keys.
my_dict = {
'age': 27,
'job': 'Youtuber',
'name': 'Digital Academy'
}
print('name' in my_dict) # True
print('salary' in my_dict) # False
print('name' in my_dict.keys()) # True
On the other hand, to check whether a certain value exists in a dictionary, you must use “in” operator, combined with the values() method.
my_dict = {
'age': 27,
'job': 'Youtuber',
'name': 'Digital Academy'
}
print('Digital Academy' in my_dict.values()) # True
print('Jérémy' in my_dict.values()) # False
Iterating over keys, values, items
Dictionaries are a very useful and widely used data structure, in Python. As a developer, you will often be in situations where you will need to iterate through a dictionary, while you perform some actions on its “key-value” pairs.
Items
When you are working with dictionaries, it is likely that you will want to work with both, the keys and the values. One of the most useful ways to iterate through a dictionary in Python, is by using items(), which is a method that returns a new view of the dictionary’s items – and will contain all of the “key-value” pairs as tuples (key, value), inside of a list. On each iteration, the current tuple will be unpacked in the form of “key, value” so you can either access the key, or the value – individually.
my_dict = {
'age': 27,
'job': 'Youtuber',
'name': 'Digital Academy'
}
for k, v in my_dict.items():
print(k, '->', v)
# OUTPUT:
# age -> 27
# job -> Youtuber
# name -> Digital Academy
# my_dict.items() = [('age', 27),('job','Youtuber'),('name', 'Digital Academy')]
Keys
If you just need to work with the keys of a dictionary, then you can use keys() function, which is a method that returns a new view object, containing the dictionary’s keys. On each iteration, the current item will only be the key – not its associated value!
my_dict = {
'age': 27,
'job': 'Youtuber',
'name': 'Digital Academy'
}
for k in my_dict:
print(k)
for k in my_dict.keys():
print(k)
# OUTPUT:
# 'age'
# 'job'
# 'name'
Values
If you use a dictionary in a for loop, it traverses the keys of the dictionary – by default. But it is also common to only use the values, to iterate through a dictionary in Python. One way to do that is to use values(), which returns a view with the values of the dictionary. On each iteration, the current item will only be the value – not its associated key!
my_dict = {
'age': 27,
'job': 'Youtuber',
'name': 'Digital Academy'
}
for k in my_dict:
print(my_dict[k])
for v in my_dict.values():
print(v)
# OUTPUT:
# 27
# 'Youtuber'
# 'Digital Academy'
Dictionary Length
Eventually, you may want to find how many “key: value” pairs a dictionary has. In that case, you do not have to iterate through a dictionary and count each item, but use the function len() – which returns the number of items in a dictionary.
my_dict = {
'age': 27,
'job': 'Youtuber',
'name': 'Digital Academy'
}
print(len(my_dict)) # OUTPUT: 3
Merging Dictionaries
You may sometimes want or need to merge two dictionaries, so that you can have all of your data located in the same variable. Or update its values, with new content. Use the built-in update() method: to merge the keys and values of one dictionary, into another one. Notice that: this method blindly overwrites values, of the same key. If there was already an existing key inside of the first dictionary, its value will be updated – with the new one. And news keys will be created, if there were not already existing. As you can see in this example, merging the two dictionaries will result in combining all of the items, and updating the ones that existed before.
my_dict1 = {
'age': 25,
'job': 'Youtuber',
'name': 'Digital Academy'
}
my_dict2 = {
'age': 27,
'city': 'Paris',
'email': 'digital.academy@free.fr'
}
my_dict1.update(my_dict2)
# my_dict1 = {'name': 'Digital Academy', 'age': 27, 'job': 'Youtuber', 'city': 'Paris', 'email': 'digital.academy@free.fr'}
Dictionary Methods
Python has a set of built-in methods that you can invoke on dictionary objects.
Method | Description |
---|---|
clear() | Removes all items from the dictionary |
copy() | Returns a shallow copy of the dictionary |
fromkeys() | Creates a new dictionary with the specified keys and values |
get() | Returns the value of the specified key |
items() | Returns a list of key:value pair |
keys() | Returns a list of all keys from dictionary |
pop() | Removes and returns single dictionary item with specified key. |
popitem() | Removes and returns last inserted key:value pair from the dictionary. |
setdefault() | Returns the value of the specified key, if present. Else, inserts the key with a specified value. |
update() | Updates the dictionary with the specified key:value pairs |
values() | Returns a list of all values from dictionary |
Python also has a set of built-in functions that you can use with dictionary objects.
Method | Description |
---|---|
all() | Returns True if all list items are true |
any() | Returns True if any list item is true |
len() | Returns the number of items in the list |
sorted() | Returns a sorted list |
Conclusion
In this tutorial, you have learned in-depth use about Dictionary, in Python. This kind of Data Types is used, when you want to store a pair of “key:value”, and access a value in a very efficient way – thanks to its key. Consequently, you should now be able to: create a dictionary, add, update or remove a few items, and even search for a specific item. Dictionary should no be a secret anymore, and you can start using them in your program, straight away!
In the next tutorial, you will discover another Data Type, in details: List! List is an ordered collection which is often use when you want to store item, in a particular order. So, do not waste any minute of it, and join us in the next episode! If you liked this video, please: do not forget to give a thumb up, and subscribe our channel!
– Digital Academy™ 🎓
📖Complete Python Development Course for Beginners
#Python #Tutorial #Beginners #Dictionary
***
♡ Thanks for watching and supporting ♡
Please Subscribe. Hit the notification bell.
Like, Comment and Share.
***
♡ FOLLOW US ♡
✧ http://digital.academy.free.fr/
✧ https://twitter.com/DigitalAcademyy
✧ https://www.facebook.com/digitalacademyfr
✧ https://www.instagram.com/digital_academy_fr
✧ https://www.youtube.com/c/DigitalAcademyOnline
***
♡ SUPPORT US ♡
✧ http://digital.academy.free.fr/join
✧ http://digital.academy.free.fr/donate
✧ http://digital.academy.free.fr/subscribe
✧ https://www.patreon.com/digital_academy
✧ https://www.buymeacoffee.com/digital_academy