Welcome back to Digital Academy, the Complete Python Development Course for Beginners.
In the previous tutorial, you may have discovered Python Conditionals: If, Else-If and Else, but also more advanced and complex decision making, so you can control the execution flow of your program. In this brand new video, you will now discover Iterations in Python, using FOR and WHILE Loops – so you can improve the execution flow of your program.
Please, Do not forget to smash that Like button, for the YouTube algorithm – since it does really help supporting us, and providing new FREE content, once a week! You may also want to follow Digital Academy, on our other social platforms: Facebook, Instagram, Twitter and Patreon. This way, we could: stay in touch, grow this community, then share and help each other, throughout this exciting journey, OUR journey! Are you interested to be part of this community? All the Links are in the description below! Now, Let’s play this video …
Definition
Loops in Python are an efficient method for optimising your code, and executing multiple statements. If the same block of code has to be executed, multiple times, over and over: you can put it into a loop, to perform multiple iterations, and get the same desired output. Consequently: it saves a lot of effort, and reduces complexity of the code, as well.
Basically, there are two types of iteration, in programming languages:
- Definite iteration: In which the number of repetitions is specified, explicitly – and in advance. This kind of iteration is performed using for loops, and has been discussed in the previous video.
- Indefinite iteration: In which the code block executes, until some condition is met. This iteration is performed using while loops, and will be discussed in the next section.
WHILE Loops
The while statement in Python, is a bit different from what you usually use, in other programming languages. Rather than iterating over a numeric progression, or over the items of an iterable, like: strings, dictionaries, lists, tuples and sets – or any other iterable objects.
While loops are traditionally used when you have a block of code which you want to repeat, indefinitely, and as long as an expression is True – or a particular condition is met. You generally use this loop when you do not know beforehand, how many iterations are going to take place.
Do not forget to contrast the while statement, with the for loop – used when you want to repeat a block of code, for a fixed number of times. Okay, But what does it look like, and how to control the execution flow of your program, using while loops? Let’s move forward, and have a look at the syntax of the while statement.
Syntax
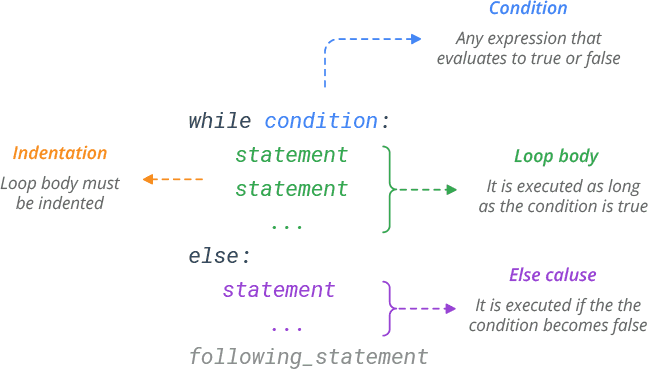
Here, is a simple declaration of while loop. First, the execution starts and checks whether the condition is True. When the condition is True, it enters the while loop – and executes the indented statements, inside the while loop. After one iteration, the condition is checked again. And this process continues until the condition evaluates to False. As soon as the condition is False, its execution skips the while loop, and moves to the next following statements in the program.
Please also note that Python interprets any non-zero value or non-empty container as True. Consequently, None, zero and empty sequence will be interpreted as False. And if the condition is False at the start, the while loop will never be executed.
Basic Example
Let’s have a more practical and very simple example, so you can discover and understand how to properly use Python while loops, in your program. First, declare an integer i, with the value of 6. Then, you will iterate and execute the block of code inside the while loop, which will print(i) and decrement the variable i. Thus, on each iteration, this expression will be evaluated again, until it becomes False – or 0, in that specific case. If you are not used to decrement a variable with this syntax (variable -= 1), please check the suggested video on the Operators, in Python.
i = 6
while i:
print(i)
i -= 1
In the above example: the execution starts with the variable i = 6. Then it tests whether the expression i is True, or i = 0. As long as the condition is True, it will enter and execute the statements – inside the loop. Until it becomes False: then it will proceed with the statements, after the loop. It is a very simple example, of how you can use while Loops in Python.
Of course, there are many other alternatives you can use, and go through any iterable object, as well. Here, is another example, when you want to iterate through every single item of a list. First, let’s declare a list of strings, and add a few strings to this list. Then, you will iterate through the list using while statement, and print each item. Very simple, Right?
colours = ['red', 'green', 'blue', 'yellow']
while colours:
colour = colours.pop()
print(colour)
The execution will start and check whether the expression is True – in that case if the list is not empty. Then, look for the first item in the sequence or any iterable object. After executing the statements in the block, which pop the current item into a variable and print it out, it will re-evaluate the condition, and the process will continue, over and over again: looks for the next item in the sequence, and print it out – until it reaches the last item of that list, yellow, and becomes empty.
Did you get it? Here is a last example, when you want to iterate through every single character of a string – because a string is a list of characters. This code will print out every single character of my_string, until the condition letter != “!” evaluates to False, or if the opposite: letter == ‘!’ becomes True.
i = 0
my_string = 'python!'
letter = my_string[i:i+1] # 1st letter of my_string
while letter != '!':
i += 1
print(letter)
letter = my_string[i:i+1] # Next letter
And here you are, you just wrote your first Python iteration, using while loops. Was not that hard? Let’s move forward to the next section, so you can alter the behaviour of your while loop – during its execution.
Break, in while Loop
In each example you have seen so far, the entire body of the while loop is executed on each iteration. It is all very well, but quite limited, Right? Fortunately, Python provides two keywords, break and continue, which terminate the current loop iteration, prematurely, without even checking the test expression. Please note that break and continue work the same way with for loops, as with while loops. Now, Let’s take a look at how you can use these control statements.

Break in Python is a control flow statement that is used to exit the execution, as soon as the break is encountered. The Python break statement, immediately terminates a loop – entirely. Consequently, the program execution then proceeds to the first statement, following the loop body.
while expression:
<statement_1>
<statement_2>
if <condition>:
break
...
<statement_3>
<following_statement>
Let’s understand how you can use a break statement in while loop, using an example. So, let’s say you have a list with strings, as items. You want to exit the loop using the break statement, as soon as the desired string is encountered.
youtube_page = ['D','I','G','I','T','A','L',' ','A','C','A','D','E','M','Y']
while youtube_page:
letter = youtube_page.pop()
if letter == ' ': break
print(letter)
In the above example, as soon as the loop encounters the space character “ ”, it will enter the if statement block where the break statement will exit the loop, straight away. Thus, it will only print DIGITAL. Did you get it?
Then let’s practice – it’s your turn now. Suppose you have a list of colours: [‘red’, ‘green’, ‘blue’, ‘yellow’], and you want to print each color, but break the loop when it encounters ‘blue’. How would you proceed? What output would you get?
colours = ['red', 'green', 'blue', 'yellow']
while colours:
colour = colours.pop()
if colour == 'blue': break
print(colour)
Here is a solution. First, Let’s declare a list, in which you added all of the colours – [‘red’, ‘green’, ‘blue’, ‘yellow’]. Then, iterate throughout this list, using while statement: while colours. It will create an iterator, and the variable colour will get the first value inside the list, on each iteration, until the execution has reached the last item in the sequence and the list becomes empty. When it encounters the item ‘blue’, you do not want to execute the code that comes next, but exit the loop – if colour == ‘blue’: break. In all the other cases, as it would be with an “else” statement, you want to print out the current colour. As you may have guessed the loop will print ‘red’, ‘green’ and stop as soon as it has encountered item blue. Congrats – You just wrote your first while loop, on your own!
Continue, in while Loop
Great! Let’s dig a little deeper, and suppose this time you do not want to exit the loop, but just skip the current iteration, and execute the rest of the iterations anyway – with the next items.
The Python continue statement, on the other hand, will immediately terminate the current loop iteration. The execution jumps to the top of the loop and re-evaluate the expression, to determine whether the loop will execute again, with the next iteration, or if it will terminate and proceed to the first statement, following the loop – like it would, with the “break” reserved keyword you have seen before.
while expression:
<statement_1>
<statement_2>
if <condition>:
continue
...
<statement_3>
<following_statement>
Let’s have a look at the same example you have seen, in the break statement. But, instead of break, you will now use the continue statement. Continue is also a control statement, but the only difference is that it will only skip the current iteration in the loop, continues with the next iteration – then executes the rest of the iterations anyway, until the while condition becomes False
colours = ['red', 'green', 'blue', 'yellow']
while colours:
colour = colours.pop()
if colour == 'blue': continue
print(colour)
In the above example, as soon as the loop encounters the item ‘blue’, it will enter the if statement block, where the continue statement will skip the current item in the loop, then continues to the next iteration, with the item yellow. This, loop will repeat until it reaches the lat item of the sequence, and result in printing out ‘red’, ‘green’ and ‘yellow’ – since blue has been skipped over.
Else, in While Loop
Good! But how can you execute some code, after the while loop statement, and still keep you code clear?
The common construct is to run a loop, and search for an item. If the item is found, we break out of the loop, using the break statement. There are two scenarios in which the loop may end. The first one is when the item is found and break is encountered. The second scenario is that the loop ends naturally, without encountering a break statement.
Now we may want to know which one of these, is the reason for a loop’s completion. One method is to set a flag, and then check, it once the loop ends. Another, is to use an optional else clause, at the end of a while loop.
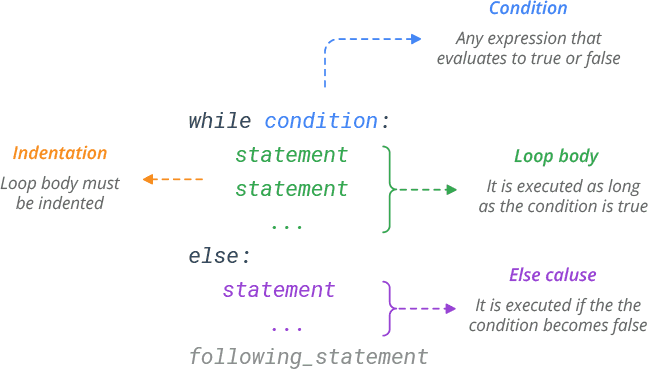
The else clause will be executed, if the loop terminates naturally – when the condition becomes False, or if it has been False since its beginning and it did not enter the loop body. On the other hand, if the loop terminates prematurely, with break, the else clause will not be executed – at all.
Here is the basic structure, of a while-else loop. First, let’s assume you declared a list, with some colours inside. Now, you want to search for a specific item – which in that case, is the colour blue. If you find it, you want to break this loop, then continue with the next statements, after the loop. But, on the other hand, if you didn’t find that item, you want to execute some code first, then and only then, execute the same code that if you found it, and proceed with the rest of your code. As you may have guessed, the function not_found_in_iterable() will never be called in this example, since the while loop will terminate prematurely – as soon as it encounters the item ‘blue’.
colours = ['red', 'green', 'blue', 'yellow']
while colours:
colour = colours.pop()
# Found it!
if colour == 'blue':
found(item)
break
else:
# Didn't find anything!
not_found_in_iterable()
always_executed()
Infinite Loop
Suppose you write a while loop that, theoretically, never ends. Sounds weird, right? Well, that kind of loop, also known as infinite loop, might be very useful in client / server programming, where the server needs to run continuously, so that the client programs can communicate with it – as and when required.
As you may have understood, an infinite while loop executes infinite times, which means: theoretically, the execution never stops. This may come as a surprise, but it has its own advantages – and disadvantages, as well. Therefore, you must use it with caution, because of the possibility that this condition never resolves to a False value – and may never exit this infinite loop.
Let’s have a look at a more practical example. This program will run infinite iterations, unless you press Ctrl+C, or put a break control statement in the loop. The condition within the while statement must eventually become False. Otherwise, the loop will execute forever – creating an infinite loop!
while True:
print('Press Ctrl+C to stop me!')
Fortunately, you can safely implement an infinite loop in your program, using the break control statement that you have seen little bit earlier. This way, as soon as the condition is met, this break keyword will immediately interrupt the current iteration, exit the infinite loop, and proceed with the next statements – outside of the loop.
while True:
name = input('Enter name:')
if name == 'stop': break
print('Hello', name)
Enter name:Alicia
Hello Alicia
Enter name:Bob
Hello Bob
Enter name:stop
Conclusion
In this tutorial, you have learned about a new structure, in Python: Infinite iterations – also known as While Loop. This is one of the statements which control the flow of execution in your program and will execute a block of code, repeatedly. You can also adapt the behaviour of the while loop, using the two control statements: break, and continue. Plus, add an additional, else clause when you want to execute some code, only if the loop terminates naturally. All of these concepts are crucial, to develop more advanced Python code – and reduce its complexity.
In the next tutorial, you will discover one of the most-used data type: Dictionary! This kind of data types is used when you want to store a pair of “key:value”, and access a value in a very efficient way, thanks to its key. So, do not waste any minute of it, and join us in the next episode! If you liked this video, please: do not forget to give a thumb up, and subscribe our channel!
– Digital Academy™ 🎓
📖Complete Python Development Course for Beginners
#Python #Tutorial #Beginners #While #Loops
***
♡ Thanks for watching and supporting ♡
Please Subscribe. Hit the notification bell.
Like, Comment and Share.
***
♡ FOLLOW US ♡
✧ http://digital.academy.free.fr/
✧ https://twitter.com/DigitalAcademyy
✧ https://www.facebook.com/digitalacademyfr
✧ https://www.instagram.com/digital_academy_fr
✧ https://www.youtube.com/c/DigitalAcademyOnline
***
♡ SUPPORT US ♡
✧ http://digital.academy.free.fr/join
✧ http://digital.academy.free.fr/donate
✧ http://digital.academy.free.fr/subscribe
✧ https://www.patreon.com/digital_academy
✧ https://www.buymeacoffee.com/digital_academy