Welcome back to Digital Academy, the Complete Python Development Course for Beginners.
In the previous tutorial, you may have discovered Python Operators: Arithmetic, Assignment, Comparison and Logical operators, but also more advanced Bitwise, Identity and Membership operators. In this brand new video, you will now discover Conditional Statements in Python, its syntax and how to properly use Logical and Comparison Operators, so you can control the execution flow of your program.
Please, Do not forget to smash that Like button, for the YouTube algorithm – since it does really help supporting us, and providing new FREE content, once a week! You may also want to follow Digital Academy, on our other social platforms: Facebook, Instagram, Twitter and Patreon. This way, we could: stay in touch, grow this community, then share and help each other, throughout this exciting journey, OUR journey! Are you interested to be part of this community? All the Links are in the description below! Now, Let’s play this video …

Everything you have seen so far, has consisted of sequential execution, in which statements are always performed, one after the next – and exactly in the order you specified, explicitly.
But the World is often more complicated than that. Frequently, a program needs to skip over some statements, execute a series of statements, repetitively – or even choose between alternate sets of statements, to execute. And this is where the core concept of Control Structure comes in, so you can control which instructions are executed, in your program.
Definition
In programming languages, most of the time you want to control the execution flow of your program, since: you want to execute some set of statements, only if the given condition is satisfied. And a different set of statements, when it is not satisfied. Please, note that Conditional Structures are also known as: Decision-making statements!

A Decision, is when a program has more than one choice of actions, depending on a variable’s value. In the real World, you commonly must evaluate information around you, and then choose one course of action or another, based on what you observe. Right?
For instance: Think of a traffic light! When it is green, you continue your drive. When you see the light turn yellow, you reduce your speed, and when it is red, you stop! These are Logical Decisions, that depend on the value of the traffic light.
Luckily, when your application needs to make such decisions in Python, the if statement is how you perform this sort of decision-making. And it allows conditional execution of a statement, or a group of statements, based on the value of a boolean expression.
If Statements
In order to write useful programs, we almost always need the ability to test conditions, and change the behaviour of the program, accordingly. Fortunately, conditional statements give us this ability.

If statements are one of the most commonly used conditional statements, in many programming languages. It decides whether certain statements need to be executed – or not. If statement checks for a given condition: when the boolean expression is True, the set of code inside the if block is executed. On the other hand: when the expression is False, the set of code inside the if block is skipped over, and not executed at all!

Now, Let’s take a look at its syntax. The boolean expression after the if statement is called the condition. A few things to keep on mind about if statements are:
- The colon (:) is significant and required. It separates the header of the compound statements, from the body.
- The line after the colon must be properly indented. In Python, it is standard to use four spaces, for indenting.
- All lines indented the same amount after the colon will be executed, whenever the Boolean expression is True.
One-Line if Statements
It is customary to write if on one line, and indented on the following line. But, if you only have a unique line of code to be executed, rather than multiple lines in the code following the condition, you can place it all in the same line. This is not a rule, but just a common practise amongst coders. Thus, both of the following are functionally equivalent:
if <expression>:
<statement>
if <expression>: <statement>
There can even be more than one statement on the same line, separated by semicolons. But what does it mean? If the expression is True, execute all of the statements. Otherwise, do not execute any of them.
if <expression>: <statement_1>; <statement_2>; ...; <statement_n>
The Python pass Statement
Occasionally, it is useful to have a section with no statements: usually as a place keeper, where you will eventually put a block of code, that you have not implemented yet.
Because Python uses indentation instead of delimiters, it is not possible to specify an empty block. If you introduce an if statement, something has to come after it: either on the same line, or indented on the following line.
To keep the interpreter happy in any situation where a statement is syntactically required, you can use the “pass” statement – which does nothing, except act as a placeholder. Here is an example:
>>> if True:
>>> pass
>>> print("After pass")
"After pass"
Example
Now that you know how to check whether a condition is True or not, Let’s get to the practice. For example, Let’s say you are recording the score for a certain course. The total score is 100, with 50 points for the theoretical work, and 50 for practical. You want to display an error message or warning, if the score exceeds 100. How would you proceed?
score_theory = 40
score_practical = 45
if(score_theory + score_practical > 100): print("Invalid score. Please check the input.")
If / Else Statements
You know how to use an if statement, to conditionally execute a single statement, or a block of several statements. It is now time to find out what else you can do, to control the execution flow of your program.
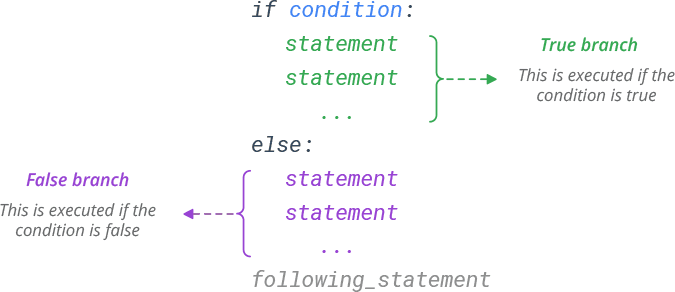
Sometimes, you want to evaluate a condition, and take one path if it is True, so that one thing or more can happen, but specify an alternative path when it is not, and something else happens. This, is accomplished with an else clause, which executes a statement in case all the tested expressions are False – in the default case.
To understand the control flow of an if-else statement, take a look at the flow chart of how the execution takes place:
if <expression>:
<statement(s)>
else:
<statement(s)>
An if expression is followed by an indented block of statements. If the expression is True: the first block of statements is executed, then the second is skipped. But, after the if statement, there is an optional else statements which is followed by another indented block of statements. When the if expression is False: the first block of statements is skipped, then, the second executed instead.
There is no limit on the number of statements that can appear under the two clauses of an if-else statement, but there has to be at least one statement in each block! Either way, execution then resumes after the second block. Also note that both suites of statements are defined by indentation, as described earlier.
Example
Great! Let’s get back and say you wanted to go a step further, in the previous example. Imagine you wanted to display a statement, when the total score was actually within the range – i.e. less than 100. ‘Cause this is exactly when the if-else statement would help.
When recording the score for a certain coursework, you want to add, together, the points for the theory part, and also the practical part to get the total score. If the total score is greater than 100, you want to display a warning message. And if it is less than 100, display a ‘Score valid’ message. Here is a solution to this problem.
score_theory = 40
score_practical = 45
if(score_theory + score_practical > 100):
print("Please check the input. Score exceeds total possible score.")
else:
print("Score validated. Your total is: ", score_theory + score_practical)
If / Elif / Else Statements
Sometimes, there are more than two possibilities, so you need more than two branches. Fortunately, there is one more conditional statements in Python, which is used to test out more expressions, and in order to optimise and increase the efficiency of your code. One way to express such a computation, is called: a chained conditionals – or else-if.
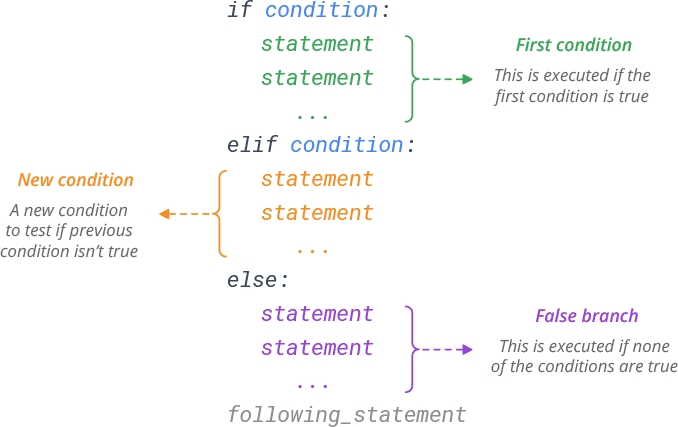
Let’s take a look at its syntax, to understand how you can declare an else-if statement block, in Python.
if <expression_1>:
<statement(s)>
elif <expression_2>:
<statement(s)>
elif <expression_3>:
<statement(s)>
...
else:
<statement(s)>
Each condition is checked in order. If the first is False: the next is checked. If the second is False: the next is checked, and so on. If one of them is True, the corresponding branch executes, and the statement ends. Even if more than one condition is True in the following statements, only the first True branch will execute.
There is no limit of the number of else-if statements, but only a single – and optional – final else statement is allowed, and it must be the last branch in the statement. If an else clause is not included and all the conditions are False, then none of the blocks will be executed.
Example
Let’s get back to our example, and imagine a case where one of these scores exceed 50, but the total is still less than 100. What do you think would happen then?
score_theory = 60
score_practical = 35
if(score_theory + score_practical > 100):
print("Please check the input. Score exceeds total possible score.")
else:
print("Score validated. Your total is: ", score_theory + score_practical)
I bet you guessed it right?? This code will output: “Score validated. Your total is: 95”. Although this is wrong, since you know that the maximum limit for individual scores for theory or practical should not exceed 50.
How can you represent this in Python code – using if-elif-else statement? One way, is to check whether the individual scores for theory and practical do not exceed 50.
score_theory = 60
score_practical = 20
if(score_theory > 50):
print("Please check the input score for 'Theory'.")
elif(score_practical > 50):
print("Please check the input score for 'Practical'.")
else:
print("Score validated. Your total is: ", score_theory + score_practical)
Nested Conditionals
When you have an if statement inside another if statement, this is called nesting, in the programming world. It does not have to always be a simple if statement, but you could use the concept of if, if-else and even if-elif-else statements, all of them combined, to form a more complex structure – and check multiple conditions, in a given code.
Although the indentation of the statements makes the structure apparent, nested conditionals become difficult to read, very quickly. In general, it is a good idea to avoid them, as much as you can, since indentation is the only way to figure out what is the level of nesting.
An if statement, present inside another if statement, which is present inside another if statements itself – and so on – is pretty difficult to read. Right? But, Let’s have a look at one example of nested conditionals:
if <expression_1>:
<statement(s)>
else:
if <expression_2>:
<statement(s)>
else:
<statement(s)>
The outer conditional contains two branches. The second branch, else, contains another if statement – which has two branches of its own. Those two branches could contain conditional statements, as well. You see now, how quickly this could become difficult to read, and even code yourself.
Conditional Expressions
Python supports one additional decision-making entity, called: a Conditional Expression, or even Ternary Operator. In its simplest form, the syntax of the Conditional Expression is as follows:
<expression_1> if <conditional_expression> else <expression_2>
This is quite different from the if statement forms, listed earlier, because it is not a control structure that directs the flow of execution of your program. It acts more like an operator, that defines an expression. In the example, this conditional expression is evaluated first. If it is True, the expression evaluates to expression 1. And if it is False, then it evaluates to the expression 2. Notice the non-obvious order: the middle expression is evaluated first, and based on that result: one of the expressions or values on the ends is returned.

As you may have guessed, a common use of the conditional expression is for variable assignment. For instance, Let’s suppose that you want to find the larger of two numbers. Of course, there is a built-in function max() that does just this – and more – that you could use. But, suppose you want to write your own code, from scratch. Then, you could use a standard if-else statement this way, or even a shorter and one-line way.
# Standard
if a > b:
m = a
else:
m = b
# One-Line
if a > b: m = a
else: m = b
But, you would agree, that a conditional expression is even shorter, and arguably more readable as well:
m = a if a > b else b
Example
Here are some other examples that will, hopefully, help you clarify this concept of conditional expressions:
>>> raining = False
>>> print("Let's go to the", 'beach' if not raining else 'library')
"Let's go to the beach"
>>> raining = True
>>> print("Let's go to the", 'beach' if not raining else 'library')
"Let's go to the library"
>>> age = 12
>>> s = 'minor' if age < 21 else 'adult'
>>> s
'minor'
>>> 'subscribe' if ('like' in ['I', 'like', 'your', 'videos']) else "like"
'subscribe'
In the very first example, raining has a boolean value of False. Thus, the expression “if not raining” is True, and results in returning the left value of the conditional expression, which is “beach”, and printing “Let’s go to the beach”! On the other hand, when raining has a boolean value of True, the conditional expression is False, which results in printing out “Let’s go to the library”! Okay? Let’s pause this video and analyse the other examples, if you want to.
Great! it is now time to practice, so: Open your favourite Code Editor, and Get to your keyboards!
Conclusion
In this tutorial, you have learned about the Conditional Statements, in Python. These are the statements which alter and control the flow of execution, in your program. All of these concepts are crucial, to develop more complex Python code. If you liked this video, please, do not forget to give a thumb up, and subscribe our channel!
In the next two tutorials, you will discover two new control structures: the while statement, and the for statement. These structures facilitate iteration: execution of a statement or block of statements, repeatedly – also known as loops. So, Do not waste any minute of it, and join us in the next episode!
– Digital Academy™ 🎓
📖 Complete Python Development Course for Beginners
#Python #Tutorial #Beginners #Conditionals #If #Else #Elif
***
♡ Thanks for watching and supporting ♡
Please Subscribe. Hit the notification bell.
Like, Comment and Share.
***
♡ FOLLOW US ♡
✧ http://digital.academy.free.fr/
✧ https://twitter.com/DigitalAcademyy
✧ https://www.facebook.com/digitalacademyfr
✧ https://www.instagram.com/digital_academy_fr
✧ https://www.youtube.com/c/DigitalAcademyOnline
***
♡ SUPPORT US ♡
✧ http://digital.academy.free.fr/join
✧ http://digital.academy.free.fr/donate
✧ http://digital.academy.free.fr/subscribe
✧ https://www.patreon.com/digital_academy
✧ https://www.buymeacoffee.com/digital_academy