Hello everyone, and Welcome back to Digital Academy – the Complete Python Development Tutorials, for Beginners.
In this brand new video, you will now discover: How to Define, Raise, Catch and Handle Exceptions in Python – with the help of a few examples. So be sure to stick around, and watch till the end, if you want to handle errors in your program, without even interrupting its execution flow!
Please, Do not forget to smash that Like button, for the YouTube algorithm – since it does really help supporting us, and providing new FREE content, once a week! You may also want to follow Digital Academy, on our other social platforms: Facebook, Instagram, Twitter and Patreon. Are you interested to be part of this community? Well, all the Links are in the description below! Now, Let’s play this video …
Definition
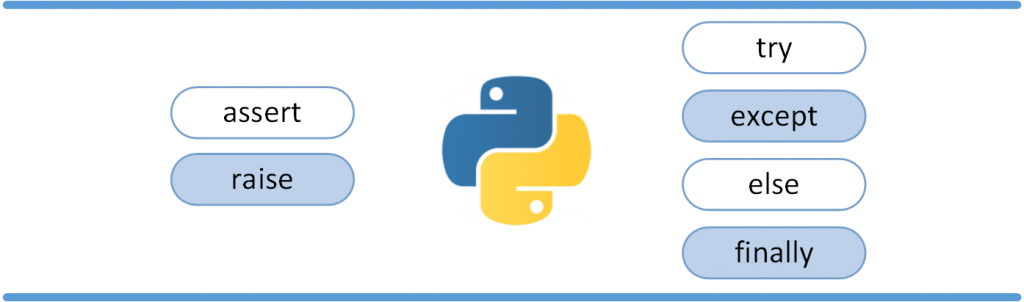
When executing Python code, different errors may occur: coding errors made by the programmer, errors due to wrong input, or even other unforeseeable errors. When your program encounters an error and that something in the program goes wrong, the Python interpreter stops the current process, and passes it to the calling process – until it is handled.
This is the default exception-handling behaviour in Python. If not handled, the program will crash straight away, and prints an error message. BUT, If you do not want this default behaviour, you need to handle these exceptions in your program. And that is where Try / Except statements comes in …
Python Errors
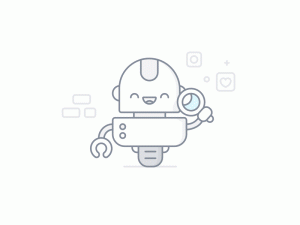
You can make certain mistakes while writing a program that lead to errors, when you try to run it. During its execution, Python program terminates as soon as it encounters an error, that is not handled. These errors can be classified, into two classes: Syntax errors, and Logical errors – also known as Exceptions.
Python Syntax Errors
Errors caused by not following the proper structure of the language, are called syntax error – or parsing error. Syntax errors are the most basic type of error. They arise when the Python parser is unable to understand a line of code. Most syntax errors are: typos, incorrect indentation, or incorrect arguments. If you get this error, try looking at your code for any of these: misspelling, missing, or misusing keyword. An arrow indicates where the parser ran into the syntax error.
for action in ["like", "comment", "share"]
^
SyntaxError: invalid syntax
Python Logical Errors (Exceptions)
Errors that occur at runtime – after passing the syntax test – are called exceptions, or logical errors. These are the most difficult type of error to find, because they will give unpredictable results and may crash your program. A lot of different things can happen, if it encounters a Logical error. Whenever these types of runtime errors occur, Python will create an Exception object. Exceptions arise when the python parser knows what to do with this piece of code, BUT is unable to perform this action. e.g trying to access the Internet without an internet connection: the Python interpreter knows what to do with that command, but is unable to perform it.
Illegal operations can raise Exceptions, too. Actually, there are plenty of built-in exceptions that are raised in Python, when the following corresponding errors occur: ValueError, TypeError, ZeroDivisionError, ImportError – and, so many more! Consequently, if not handled properly, Python prints the Traceback of that error. And the last line of this message indicates what type of exception error your program ran into – along with some details about what triggered this error.
for i in [1, 2, 3]:
print(i / 0)
Traceback (most recent call last):
File "<stdin>", line 2, in <module>
ZeroDivisionError: division by zero
Fortunately, you can catch and handle all these kind of exceptions, then adapt your program so it does not crash. You can also define your own exceptions in Python, when required! After seeing the differences between Syntax errors and Exceptions, Let’s move forward and see multiple ways to raise, catch, and handle these Exceptions, in Python.
Python Assertion

Instead of waiting for a program to crash midway, you can also start by making an assertion in Python, and verify that a certain condition is met. If this condition turns out to be True, then that is excellent – your program continues. But, if the condition turns out to be False, your program will throw an AssertionError Exception.
Let’s have a look at the following example, where it is asserted that the code will be executed on a Linux system:
import sys
assert ('linux' in sys.platform), "This code runs on Linux only."
If you run this code on a Linux machine, the assertion passes. If you were to run this code on a Windows machine, or any other machine, the outcome of the assertion would be False and the result would be the following:
Traceback (most recent call last):
File "<input>", line 2, in <module>
AssertionError: This code runs on Linux only.
In this example, throwing an AssertionError exception is the last thing that the program will do. The program will come to halt, and will not continue. BUT, What if that is not what you want?! Then, you will have to catch this Exception…
Python Try … Except
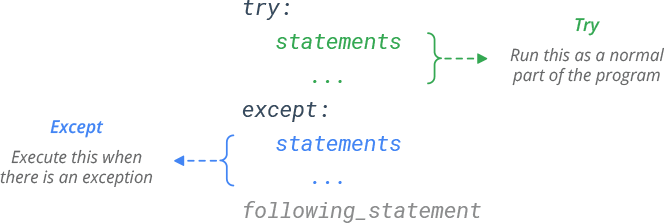
In Python, All exceptions can be handled using the “try/except” statements. The critical operations which can raise an exception is placed inside the try clause. And the code that handles the exception is written in the except clause. You can thus choose what operations to perform, once you have caught this exception. Now, Let’s have a closer look…
Catch ALL Exceptions
When an error occurs: Python would normally throw an exception, generate an error message and stop the program, if this exception is not handled properly. BUT, when this error occurs during its execution – and inside the try statement – the rest of the try block is skipped as soon as this error is encountered, and the except block is executed.
try:
statement_1
statement_fail
statement_not_executed
except:
print('Something went wrong!')
That’s right: the very simple try and except block in Python is used to catch and handle ALL these exceptions. Python will execute the code following the try statement, until an exception is encountered. The code that follows the except statement determines how your program responds to ANY of these exceptions. Consequently, the except clause is used to catch and handle ALL the exceptions that are encountered in the try clause.
What you did not get to see though, was the type of error that was thrown as a result of the exception. In order to see exactly what went wrong, you would need to catch the error that the function threw, with an alias (except Exception AS error).
try:
statement_1
statement_fail
statement_not_executed
except Exception as error:
print('Something went wrong:', error)
Awesome, but what if you do not want to execute the same block of code, for every exceptions?? Fortunately for you, Python provides the ability to catch a single and specific exception, or even multiple exceptions at once. Let’s find out
Catch Single Exception
In the previous example, we did not mention any specific exception in the except clause. It is not a good programming practice, as it will catch ALL exceptions regardless of your error, and handles every case in the same way. Nonetheless, you can specify which exceptions an except clause will catch: ValueError, TypeError, ZeroDivisionError, ImportError, etc.
try:
x = 1/0
statement_not_executed
except ZeroDivisionError:
print('Something went wrong!')
In above example, the try block will generate an exception, because a number is divided by zero. Therefore, the except block ZeroDivisionError will be executed ONLY for this specific error – any other errors will not be handled in this case, and may end up crashing your program.
That’s great, but you are wondering: How to handle multiple exceptions in Python then? Here is the solution …
Catch Multiple Exceptions
A try clause can have any number of except clauses, to handle different exceptions. However only one will be executed in case an exception occurs. Consequently, you can define as many except blocks as you want – so that you can catch and handle specific exceptions individually, and differently.
try:
x = 1/0
except ZeroDivisionError:
print('Attempt to divide by zero')
except:
print('Something else went wrong')
And, if you want to execute the same block of code, for multiple exceptions, specify ALL the exceptions inside a tuple:
try:
x = 1/0
except TypeError:
print('TypeError is raised')
except (ZeroDivisionError, ValueError):
print('ZeroDivisionError or ValueError is raised')
except:
print('Something else went wrong')
Please, also note that: Python does not just handle exceptions if they occur immediately in the “try” block, but also if they occur inside functions – that are called in the try block. And, when an error occurs, but you do not know how to properly handle it, you can use the reserved keyword “pass”, so that your program does not crash – then continues.
def division_by_zero():
x = 1/0
try:
division_by_zero()
except Exception as e:
print('Attempt to divide by zero')
pass
next_statement_executed
That is so cool. Your program will never crash anymore, since you can catch and handle all exceptions raised during its execution! But, How would you execute a block of code, only when no exceptions were encountered in the try clause?
Python Else Clause
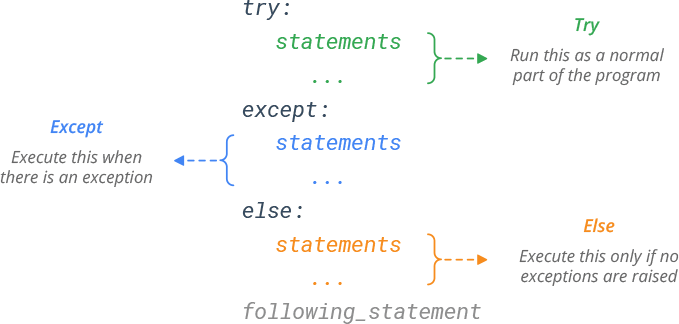
In some situations, you might want to run a certain block of code, if the code inside the try clause ran without any errors. For these cases, you can use the optional else keyword, with the try statement, to define a block of code to be executed, if and only if no errors were raised.
Note: Exceptions in the else clause are not handled by the preceding except clauses.
try:
x = 1/1
except:
print('Something went wrong')
else:
print('Nothing went wrong')
Here is an example that will divide the integer 1 by 1 – and store its result in the variable x. If an error occurs during this process, an exception is raised, then the except clause will catch and handle this error – printing out ‘Something went wrong’. Else, it will print out ‘Nothing went wrong’ because the program did not run into any exceptions, and the else clause was executed.
That is great! You can catch exceptions, handle them properly, and execute some code when no exceptions are raised. But, How would you execute a block of code even though an error occurred? Let’s find out throughout the next section
Python Finally Clause

The try…except statement in Python has another and optional finally clause. The finally block, if specified, will always be executed – regardless if the try block raises an error or not, and under any circumstances.
try:
x = 1/0
except:
print('Something went wrong')
finally:
print('Always execute this')
Imagine that you always have to implement some sort of action to clean up after executing your code. Well, Python allows you to do so, using the finally clause. This can be very useful to: close objects, release and clean up external resources.
For instance, you may be connected to a remote data center through the network, or working with a file… In all these circumstances, you must clean up the resource before the program comes to a halt, whether it successfully ran or not. All these actions (closing a file or disconnecting from network) are performed only in the finally clause, to guarantee its execution. Therefore, the program can continue properly – without leaving the file object open.
f = open('myfile.txt')
try:
print(f.read())
except:
print("Something went wrong")
finally:
f. close()
Raising an Exception
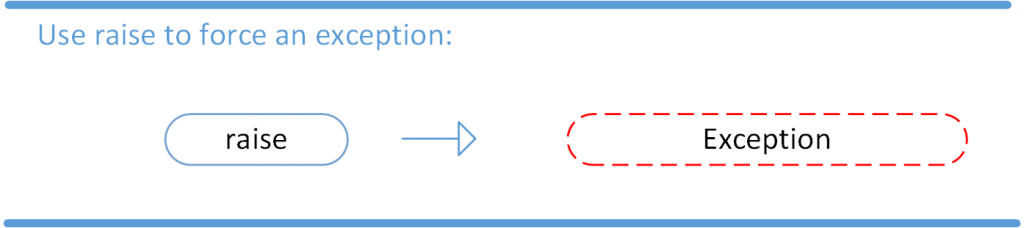
In Python programming, exceptions are raised when errors occur at runtime. But, as a Python developer, you can also choose to manually throw an exception, if a condition is met – at any time. To raise such an exception, use the “raise” reserved keyword.
This statement can be complemented with a custom exception. You can optionally define what kind of error to raise, and pass values to the exception, to clarify why that exception was raised. Then the program comes to a halt, and it displays your exception to screen – offering clues about what went wrong and help debugging.
# Raise built-in exception 'NameError'
raise NameError('An exception occured!')
# Output:
# Traceback (most recent call last):
# File "<stdin>", line 1, in <module>
# NameError: An exception occured!
User-defined Exceptions
In Python, users can define custom exceptions by creating a new class. This exception class has to be derived, either directly or indirectly, from the built-in Exception class. Most of the built-in exceptions are also derived from this class.
Here, we have created a user-defined exception called CustomError, which inherits from the Exception class. This new exception, like any other exceptions, can be raised using the raise statement – and with an optional error message.
class CustomError(Exception):
pass
raise CustomError
raise CustomError("A custom error occurred")
# Traceback (most recent call last):
# File "<stdin>", line 4, in <module>
# CustomError: A custom error occurred
… And that’s it, you can now define your own exceptions and handle them individually, in a very different way …
Conclusion
In this complete Python Tutorial for Beginners you have discovered Exceptions in Python, so that you can: define, raise, catch and handle these Exceptions in Python. Therefore, you should now be able to catch these Errors in your program – without even interrupting its execution flow. Consequently, Exceptions in Python should not be a secret anymore, and you can start using them in your Python program, straight away!
In the next tutorial, you will finally discover: File handling in Python. So, do not waste any minute, and join us in the next episode! If you liked this video, Please: Do not forget to give a Thumb up, and Subscribe our channel!
– Digital Academy™ 🎓
📖 Complete Python Development Course for Beginners
#Python #Tutorial #Beginners #Exceptions
***
♡ Thanks for watching and supporting ♡
Please Subscribe. Hit the notification bell.
Like, Comment and Share.
***
♡ FOLLOW US ♡
✧ http://digital.academy.free.fr/
✧ https://twitter.com/DigitalAcademyy
✧ https://www.facebook.com/digitalacademyfr
✧ https://www.instagram.com/digital_academy_fr
✧ https://www.youtube.com/c/DigitalAcademyOnline
***
♡ SUPPORT US ♡
✧ http://digital.academy.free.fr/join
✧ http://digital.academy.free.fr/donate
✧ http://digital.academy.free.fr/subscribe
✧ https://www.patreon.com/digital_academy
✧ https://www.buymeacoffee.com/digital_academy