Welcome back to Digital Academy, the Complete Python Development Course for Beginners.
In the previous tutorial, You may have discovered Variables and Data Types, from the very first moment it has been created, throughout its entire lifetime, as well as creating and naming Python objects of different Data Types. In this new video, you will now discover multiple Operators in Python, and How to use them.
Please, Do not forget to smash that Like button, for the YouTube algorithm – since it does really help supporting us, and providing new FREE content, once a week! You may also want to follow Digital Academy, on our other social platforms: Facebook, Instagram, Twitter and Patreon. This way, we could: stay in touch, grow this community, then share and help each other, throughout this exciting journey, OUR journey! Are you interested to be part of this community? All the Links are in the description below! Now, Let’s play this video …
Python language is one of the most popular programming languages. Whilst learning Python is seemingly easy, there are certain core concepts that must be mastered, before moving on with various applications of Python. Operators in Python are one of the core fundamental concepts. Therefore, this video will help you understand the different types of Operators in Python, so let’s stick around and watch until the end of this video!
What Is An Operator?
Operators in Python are special symbols you will use for operations between two values, or variables. The values that an operator acts on, are called operands. And the output varies according to the type of operator you have used in the operation.
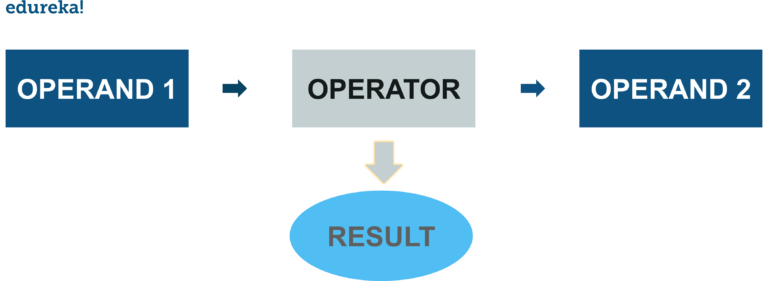
Suppose if you want to perform addition of two variables or values, you can use the addition operator for this special operation. The values in the operands can be variable, or any data type that you have in Python. Here is an example:
>>> a = 5
>>> b = 9
>>> a + b
14
In this case, the “+” operator adds the operands a and b together. An operand can be either a literal value, or a variable that references an object. A sequence of operands and operators, like “a + b”, is called an expression. Python supports many operators, for combining data objects into expressions. These operators are explored in the next section.
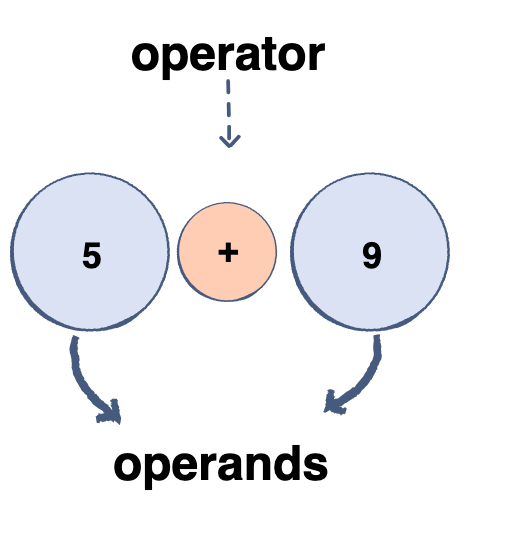
Types of Operators
Depending upon the type of operations you want to perform, there are 7 types of operators in Python.
- Arithmetic operators
- Assignment operators
- Comparison operators
- Logical operators
- Bitwise operators
- Identity operators
- Membership operators
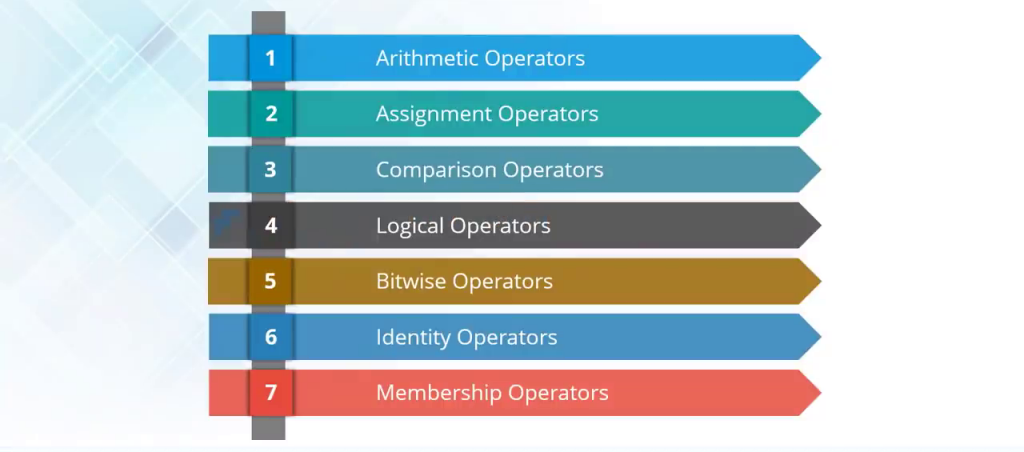
Arithmetic Operators
Arithmetic operators are used to perform common mathematical operations in Python, like: addition, subtraction and multiplication, but also division – among so many others. Below are the Arithmetic Operators with symbols, and their meaning. These are simple mathematical operators that you will use, while doing an arithmetic operation in Python.

Apart from these four basic Arithmetic Operators, you also have exponential, modulus and floor division operators:
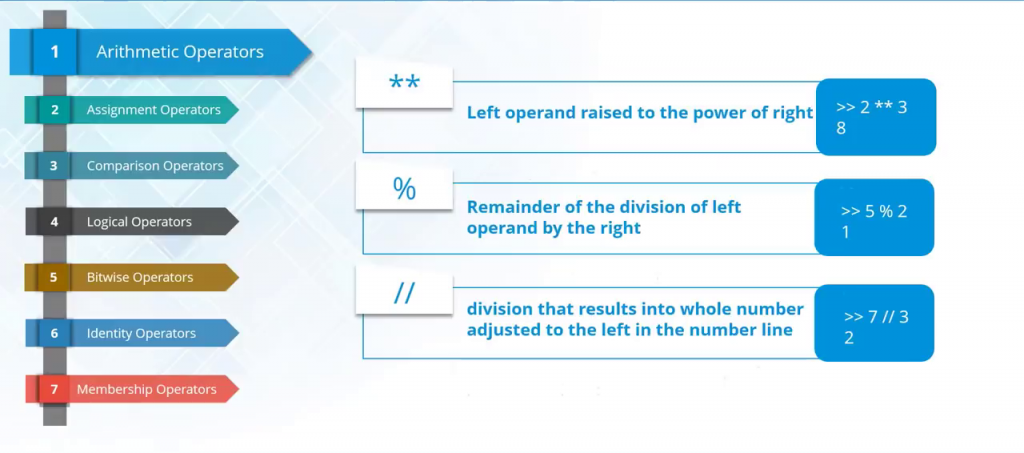
# Output: x ** y = 5062
print('x ** y =', x**y)
# Output: x % y = 3
print('x % y =', x%y)
# Output: x // y = 3
print('x // y =', x//y)
Please note that the result of standard division (/) is always a float, even if the dividend is evenly divisible by the divisor. Whereas the fractional portion of floor division (//) is truncated off, leaving only the integer portion.
>>> 10 / 5
2.0
>>> type(10 / 5)
<class 'float'>
>>> 10 // 4
2
>>> type(10 // 4)
<class 'int'>
Assignment Operators
Assignment operators are used to assign values to the variables, or any other object in python. For instance, x = 5 is a simple assignment operator, that assigns the value 5 (on the right) to the variable x (on the left). There are also multiple compound operators in Python, like: x += 5, that adds to the variable and later assigns the same. Therefore, this is the equivalent of: x = x + 5.
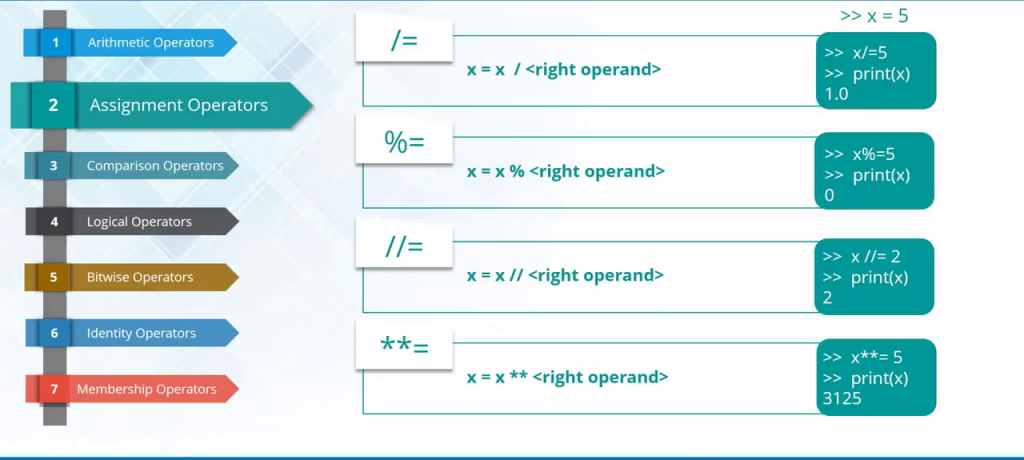
Comparison Operators
Next, Let’s move forward to the next Operator: Comparison Operators, which are used to compare 2 values. Since it either returns True or False, comparison operators are typically used in Boolean contexts – like Conditional and Loop statements, in order to direct program flow – as you will see later, in the upcoming video!
Following are the Comparison Operators that we have in Python: greater than, lesser than, equal to, and not equal to.
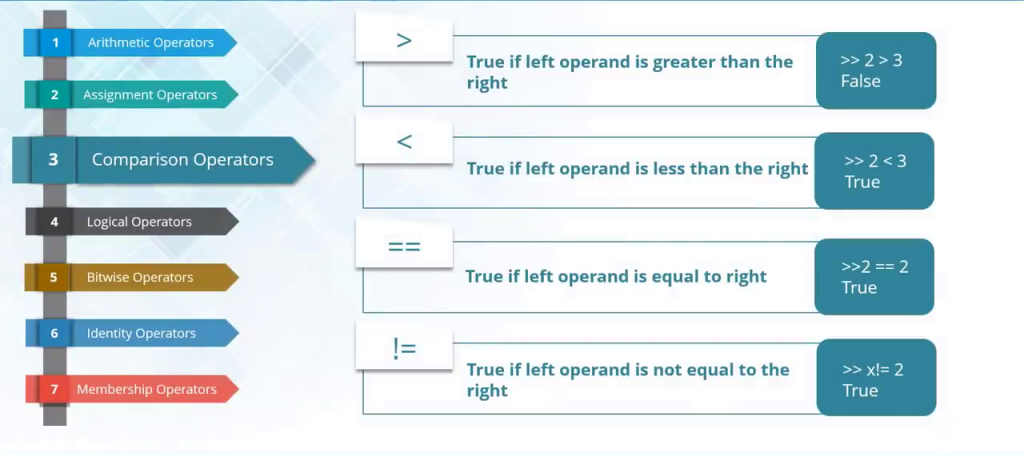
x = 10
y = 12
# Output: x > y is False
print('x > y is', x>y)
# Output: x < y is True
print('x < y is', x<y)
# Output: x == y is False
print('x == y is', x==y)
# Output: x != y is True
print('x != y is', x!=y)
# Output: x >= y is False
print('x >= y is', x>=y)
# Output: x <= y is True
print('x <= y is', x<=y)
Logical Operators
Logical operators, “and”, “or”, and “not” operators are used to compare two conditional statements. The Logical Operators modify and join together expressions, evaluated in Boolean context, to create more complex conditions.
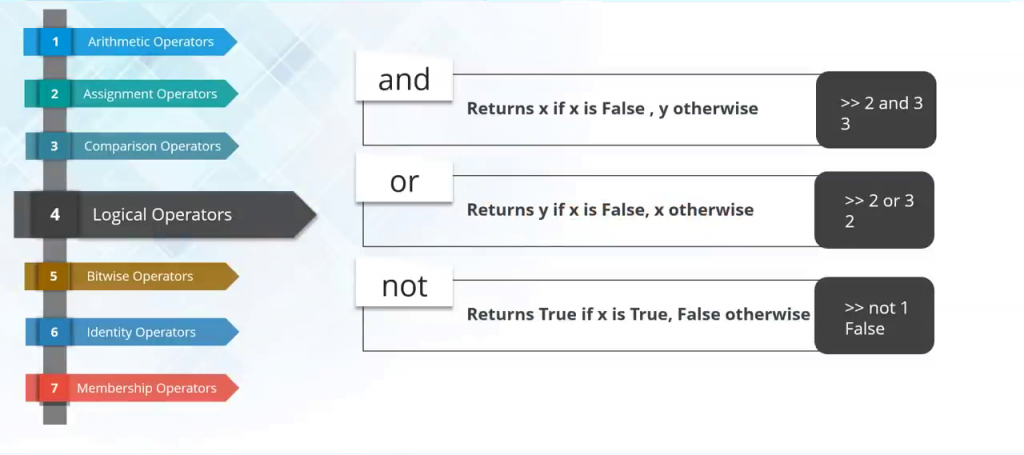
When you use the “and” operator: The expression will return True, if both operands are True. Whereas using the “or” operator will only result in True, if at least one of the operands is True. Finally, the “not” operator will only give True, if the boolean expression as the operand is False.
x = 5
# Output: x < 1O is True
print('x < 10 is', x < 10)
x = True
y = False
# Output: x and y is False
print('x and y is', x and y)
# Output: x or y is True
print('x or y is', x or y)
# Output: not x is False
print('not x is', not x)
Bitwise Operators
Let’s move forward and look at Bitwise Operators, which are similar to Logical Operators but they treat operands as sequences of Binary digits, and operate on them bit by bit. For example, 5 is 101 in binary form, and 7 is 111.
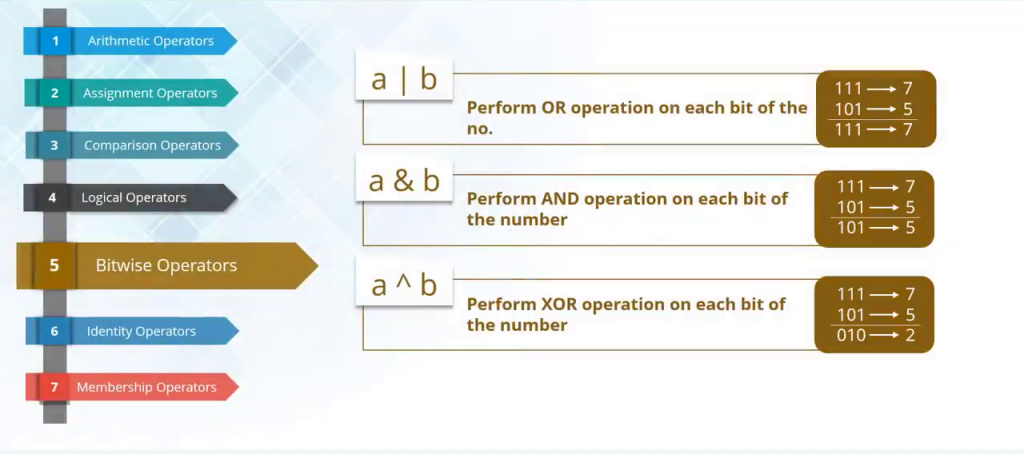
When you perform a OR (|) operation on these operands, it will give you the output 7 (111, in binary form), since you performed a Logical operation on each bit of the numbers, one at a time! This is exactly the same, when you perform the AND (&) operation, but using the appropriate logical operation, bit by bit.
Finally but not least, there is an other bitwise operator in Python, called XOR (^). It performs the exclusive OR operation on each bit of the numbers, and will return True only if one bit is True – not the both of them!
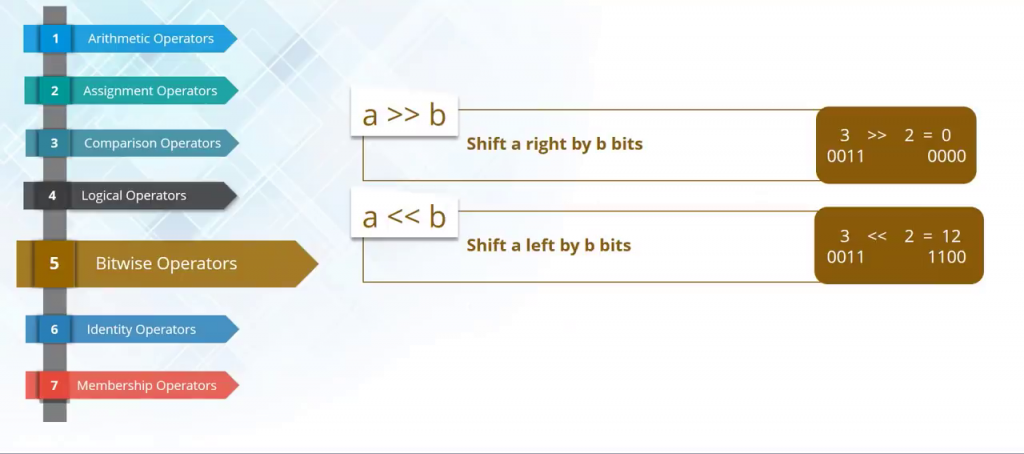
Apart from these three operators, you have two more bitwise operators, which are right shift, and left shift operators.
What basically happens, when this type of operand is used? First, the value is converted into its binary representation. Then, you will have to specify which type of shift you want to perform, using two greater than signs, or two lesser than signs. Finally, Once you have done that, you will have to specify the number of bits you want to shift! Then, the binary output will shift towards the direction you chose, left or right, for the number of bits you specified.
Identity Operators
Python language offers some special type of operators, like the Identity Operator or the Membership Operator. They are described in the next sections, with a few examples for each one of them.
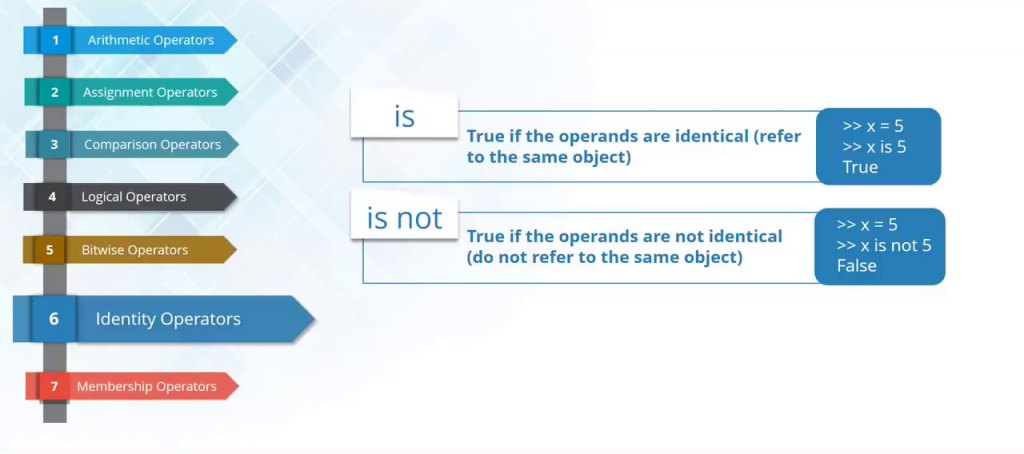
Identity Operators, is and is not, are used to compare two values, or variables: not if they are equal, but if they are actually the same Object, located on the same part of the memory. Hence, the 2 variables that are equal does not necessarily imply that they are identical — with de same identity!
Here is an example of 2 objects that are equal, but not identical. x and y both refer to objects whose value is 5: they are equal. But they do not reference the same object, as you can verify: x and y do not have the same identity, and “x is y” returns False.
>>> x = 5
>>> y = 4 + 1
>>> print(x, y)
5 5
>>> x == y
True
>>> x is y
False
You saw previously that when you make an assignment like “x = y”, Python merely creates a second reference, to the same object – and you could confirm that fact with the id() function. You can also confirm it, using the “is” operator.
In this specific case, since a and b reference the same object, it stands to reason that a and b would be equal, as well.
>>> a = "I am a string"
>>> b = a
>>> id(a)
55993992
>>> id(b)
55993992
>>> a is b
True
>>> a == b
True
Unsurprisingly, the opposite of “is”, is “is not”:
>>> x = 5
>>> y = 4 + 1
>>> x is not y
True
Membership Operators
Membership Operators, “in” and “not in”, are used to test whether a value, or variable, is found in a sequence: String, List, Tuple, Set or Dictionary. Following are the Membership Operators that we have in Python, and return a boolean.
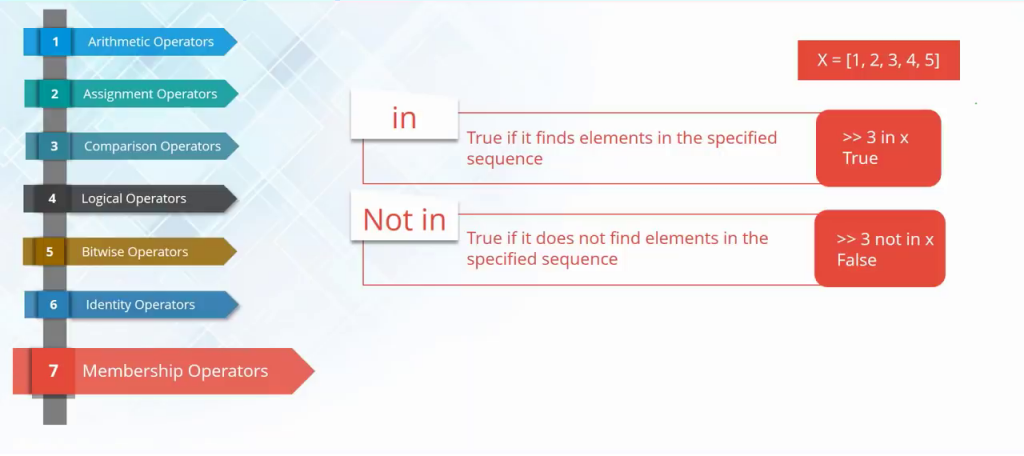
For instance, if a value 3 is found in the list X=[1, 2, 3, 4, 5], the statement “3 in X” will return True. On the other hand, the statement “3 not in X” will return False, since 3 is not an element of this list. Following are a few examples:
x = 'Hello world'
y = {1:'a', 2:'b'}
# Output: True
print('H' in x)
# Output: True
print('hello' not in x)
# Output: True
print(1 in y)
# Output: False
print('a' in y)
Operator Precedence
You now have a complete overview of the different operators in Python, but keep in mind that: When two operators share an operand, the operator with the higher precedence goes first.

For instance, consider this following expression. Since multiplication has a higher precedence than addition, “a + b * c” is treated as a + (b * c), and a * b + c is treated as (a * b) + c.
>>> 20 + 4 * 10
60
There is ambiguity here. Should Python perform the addition 20 + 4 first, and then multiply the sum by 10? Or should the multiplication 4 * 10 be performed first, and the addition of 20 only then?
Clearly, since the result is 60, Python has chosen the latter; if it had chosen the former, the result would be 240. This is standard algebraic procedure, found universally in virtually all programming languages.
Consequently, all operators that the language supports, are assigned a precedence. In an expression, all operators of highest precedence are performed first. Once those results are obtained, operators of the next highest precedence are performed. So it continues, until the expression is fully evaluated. Any operators of equal precedence are performed in left-to-right order.
Here is the order of precedence of the Python operators you have seen so far, from lowest to highest. Operators at the bottom of the table have the lowest precedence, and those at the top of the table have the highest. Any operators in the same row of the table have equal precedence.
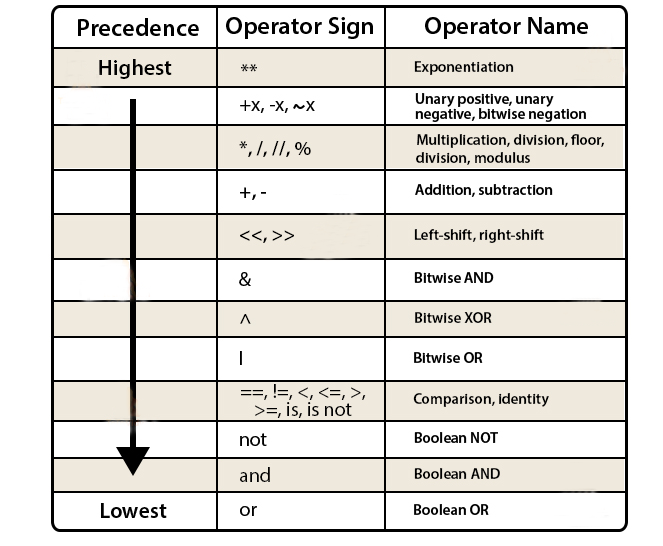
It is clear why multiplication is performed first in the example above: multiplication has a higher precedence than addition.
Similarly, in the example below, 3 is raised to the power of 4 first, which equals 81, and then the multiplications are carried out in order from left to right (2 * 81 * 5 = 810):
>>> 2 * 3 ** 4 * 5
810
Operator precedence can be overridden using parentheses. Expressions in parentheses are always performed first, before expressions that are not parenthesised. Thus, the following happens:
>>> 20 + 4 * 10
60
>>> (20 + 4) * 10
240
>>> 2 * 3 ** 4 * 5
810
>>> 2 * 3 ** (4 * 5)
6973568802
In the first example, 20 + 4 is computed first, then the result is multiplied by 10. In the second example, 4 * 5 is calculated first, then 3 is raised to that power, then the result is multiplied by 2.
There is nothing wrong with making liberal use of parentheses, even when they are not necessary to change the order of evaluation. In fact, it is considered good practice, because it can make the code more readable, and it relieves the reader of having to recall operator precedence from memory.
Consider the following:
(a < 10) and (b > 30)
Here the parentheses are fully unnecessary, as the comparison operators have higher precedence than and does and would have been performed first anyhow. But some might consider the intent of the parenthesised version more immediately obvious than this version without parentheses:
a < 10 and b > 30
On the other hand, there are probably those who would prefer the latter; it is a matter of personal preference. The point is, you can always use parentheses if you feel it makes the code more readable, even though they are not necessary to change the order of evaluation.
Conclusion
In this tutorial, you discovered: multiple operators that Python supports, so you can combine objects into expressions. You should now have quite a good understanding of the assignment, logical and comparison operators. This way, you are fully prepared to explore Conditions in much more detail, so you can now control the execution flow of your Python program, If … Else statements, which will be covered in the very next upcoming video. So, Do not waste any minute of it, and join us in the next episode!
– Digital Academy™ 🎓
📖Complete Python Development Course for Beginners
#Python #Tutorial #Beginners #Operators
***
♡ Thanks for watching and supporting ♡
Please Subscribe. Hit the notification bell.
Like, Comment and Share.
***
♡ FOLLOW US ♡
✧ http://digital.academy.free.fr/
✧ https://twitter.com/DigitalAcademyy
✧ https://www.facebook.com/digitalacademyfr
✧ https://www.instagram.com/digital_academy_fr
✧ https://www.youtube.com/c/DigitalAcademyOnline
***
♡ SUPPORT US ♡
✧ http://digital.academy.free.fr/join
✧ http://digital.academy.free.fr/donate
✧ http://digital.academy.free.fr/subscribe
✧ https://www.patreon.com/digital_academy
✧ https://www.buymeacoffee.com/digital_academy