Welcome back to Digital Academy, the Complete Python Development Course for Beginners.
In the previous tutorial, you may have discovered Python Conditionals: If, Else-If and Else, but also more advanced and complex decision making, so you can control the execution flow of your program. In this brand new video, you will now discover Iterations in Python, using FOR and WHILE Loops – so you can improve the execution flow of your program.
Please, Do not forget to smash that Like button, for the YouTube algorithm – since it does really help supporting us, and providing new FREE content, once a week! You may also want to follow Digital Academy, on our other social platforms: Facebook, Instagram, Twitter and Patreon. This way, we could: stay in touch, grow this community, then share and help each other, throughout this exciting journey, OUR journey! Are you interested to be part of this community? All the Links are in the description below! Now, Let’s play this video …
Definition
Loops in Python are an efficient method for optimising your code, and executing multiple statements. If the same block of code has to be executed, multiple times, over and over: you can put it into a loop, to perform multiple iterations, and get the same desired output. Consequently: it saves a lot of effort, and reduces complexity of the code, as well.
Basically, there are two types of iteration, in programming languages:
- Indefinite iteration: In which the code block executes, until some condition is met. This iteration is performed using while loops, and will be discussed in the very next upcoming video.
- Definite iteration: In which the number of repetitions is specified, explicitly – and in advance. This kind of iteration is performed using for loops, and will be discussed in the next section.
FOR Loops
The for statement in Python, is a bit different from what you usually use, in other programming languages. Rather than iterating over a numeric progression, Python’s for statement iterates over the items of an iterable: strings, dictionaries, lists, tuples and sets – or any other iterable objects.
For loops are traditionally used when you have a block of code which you want to repeat, a fixed number of times. The Python for statement iterates over the members of a sequence, then executes the same block each time, and in order.
Do not forget to contrast the for statement with the while loop, used when the condition needs to be checked for every single iteration, or to repeat a block of code – forever.
Okay, But what does it look like, and how to control the execution flow of your program, using for loops? Let’s move forward, and have a look at the syntax of the for statement.
Syntax
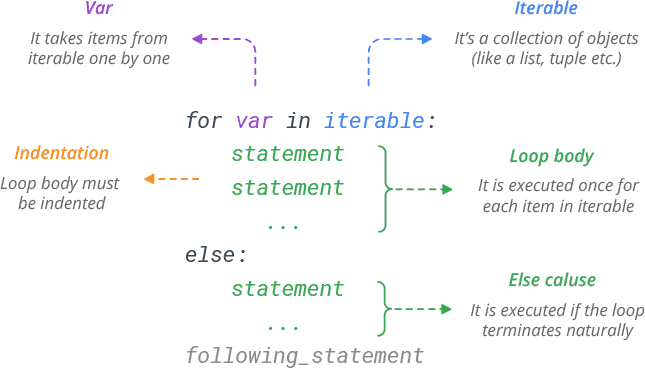
Here, is a simple declaration of for loop, in which the variable “var” takes the value of an item, inside the sequence, and on each iteration. Loop executes all statements and it continues, until we reach the last item of the sequence. The body of loop, which includes some statements, is separated from the rest of the code, using indentation.

This loop can be described entirely in terms of the concepts you have just learned about. Thus, in order to carry out the iteration this for loop describes, Python does the following steps:
- Calls iter(), to obtain an iterator for the iterable ;
- Calls next() repeatedly, to obtain each item from the iterator ;
- Executes the loop body: once for each item, and set the variable to the given item ;
- Terminates the loop, when next() raises the StopIteration exception, then continues.
Basic Example
Let’s have a more practical and very simple example, so you can discover and understand how to properly use Python for loops, in your program. First, declare a list of strings, and add a few strings to this list. Then, you will iterate through this list using for statement, and print each item. Very simple, Right?
colours = ['red', 'green', 'blue', 'yellow']
for colour in colours:
print(colour)
The execution will start and look for the first item in the sequence or iterable object. It will check whether it has reached the end of the sequence or not. After executing the statements in the block, it will look for the next item in the sequence and the process will continue, over and over again, until the execution has reached the last item in the sequence.
In the above example, the execution started from the first item in the list colours, and it went on until the execution has reached its last item – which is yellow. This is a very simple example of how you can use for Loops, in Python.
Of course, there are many other alternatives you can use, and go through any iterable object. Here is another example, when you want to iterate through every single character of a string – because a string is a list of character, remember?
my_string = 'python'
for letter in my_string:
print(letter)
And here you are, you just wrote your first Python iteration, using for loops. This very simple code will iterate through a list, and print out every single item of that list. Was not that hard? Let’s move forward to the next section!
In each example you have seen so far, the entire body of the for loop is executed, on each iteration. It is all very well, but quite limited, Right? Fortunately, Python provides two keywords, that will terminate a loop iteration, prematurely.

Break, in for Loop
Break in python is a control flow statement that is used to exit the execution, as soon as the break is encountered. The Python break statement, immediately terminates a loop – entirely. Consequently, the program execution then proceeds to the first statement, following the loop body.
for var in sequence:
<statement_1>
<statement_2>
if <condition>:
break
...
<statement_3>
<following_statement>
Let’s understand how you can use a break statement in for loop, using an example. So, let’s say you have a list with strings, as items. You want to exit the loop using the break statement, as soon as the desired string is encountered.
youtube_page = ['D','I','G','I','T','A','L',' ','A','C','A','D','E','M','Y']
for letter in youtube_page:
if letter == ' ':
break
print(letter)
In the above example, as soon as the loop encounters the space character “ ”, it will enter the if statement block where the break statement will exit the loop, straight away. Thus, it will only print DIGITAL. Did you get it?
Then let’s practice – it’s your turn now. Suppose you have a list of colours: [‘red’, ‘green’, ‘blue’, ‘yellow’], and you want to print each colour, but break the loop when it encounters ‘blue’. How would you proceed? What output would you get?
colours = ['red', 'green', 'blue', 'yellow']
for colour in colours:
if colour == 'blue':
break
print(colour)
Here is a solution. First, Let’s declare a list, in which you added all of the colours – [‘red’, ‘green’, ‘blue’, ‘yellow’]. Then, iterate throughout this list, using for statement: for colour in colours. It will create an iterator, and the variable colour will get the next value, on each iteration, until the execution has reached the last item in the sequence. When it encounters the item ‘blue’, you do not want to execute the code that comes next, but exit the loop – if colour == ‘blue’: break. In all the other cases, as it would be with an “else” statement, you want to print the current colour. As you may have guessed the loop will print ‘red’, ‘green’ and stop – because the next item is blue. Congrats – You just wrote your first for loop, on your own!
Continue, in for Loop
Great! Let’s dig a little deeper, and suppose this time you do not want to exit the loop, but just skip the current iteration, and execute the rest of the iterations anyway – with the next items.
The Python continue statement on the other hand, will immediately terminate the current loop iteration. The execution jumps to the top of the loop and re-evaluate the expression, to determine whether the loop will execute again, with the next iteration, or if it will terminate and proceed to the first statement, following the loop – like it would, with the “break” reserved keyword you have seen before.
for var in sequence:
<statement_1>
<statement_2>
if <condition>:
continue
...
<statement_3>
<following_statement>
Let’s have a look at the same example you have seen, in the break statement. But, instead of break, you will now use the continue statement. Continue is also a control statement, but the only difference is that it will only skip the current iteration in the loop, continues with the next iteration – then executes the rest of the iterations anyway, until it reaches the last item of the sequence.
colours = ['red', 'green', 'blue', 'yellow']
for colour in colours:
if colour == 'blue':
continue
print(colour)
In the above example, as soon as the loop encounters the item ‘blue’, it will enter the if statement block, where the continue statement will skip the current item in the loop, then continues to the next iteration, with the item yellow. This, will result in printing out ‘red’, ‘green’ and ‘yellow’ – since blue has been skipped over.
Else in for Loop
Good! But how can you execute some code, after for loop statement, and still keep you code clear?
The common construct is to run a loop, and search for an item. If the item is found, we break out of the loop, using the break statement. There are two scenarios in which the loop may end. The first one is when the item is found and break is encountered. The second scenario is that the loop ends without encountering a break statement. Now we may want to know which one of these, is the reason for a loop’s completion. One method is to set a flag, and then check, it once the loop ends. Another, is to use an optional else clause, at the end of for loop.
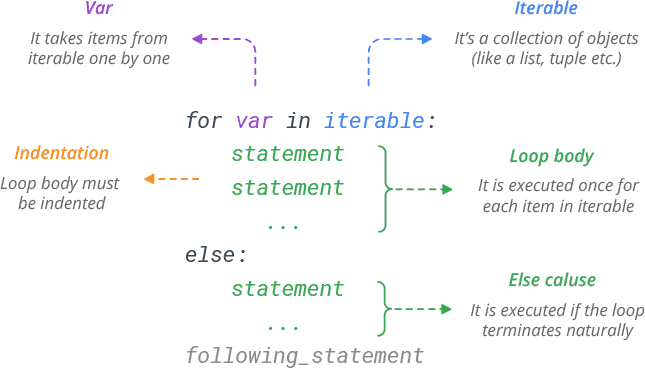
The else clause will be executed, if the loop terminates naturally – through exhaustion of the iterable object. On the other hand, if the loop terminates prematurely, with break, the else clause will not be executed – at all.
Here is the basic structure, of a for-else loop. First, let’s assume you declared a list, with some colours inside. Now, you want to search for a specific item – which in that case, is the colour blue. If you find it, you want to break this loop, then continue with the next statements after the loop. But on the other hand, if you didn’t find that item, you want to execute some code first, then and only then, execute the same code that if you found it, and proceed with the rest of your code.
colours = ['red', 'green', 'blue', 'yellow']
for colour in colours:
if colour == 'blue':
# Found it!
found(item)
break
else:
# Didn't find anything!
not_found_in_iterable()
always_executed()
Nested for Loop
Loops can be nested in Python, as they can with other programming languages. The program first encounters the outer loop, executing its first iteration. This first iteration triggers the inner, nested loop, which then runs to completion. Thus, the “inner loop” will be executed one time, for each iteration of the “outer loop”.
my_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
for sub_list in my_list:
for number in sub_list:
print(number)
range() function
Awesome! You are now able to write your own iterations – then repeat a block of code, until you have reached the last item of a list. But, in most cases, you would like to execute a group of statements, for a fixed number of times. Right?

Fortunately, Python provides a very simple way to repeat an action, for a specific number of times: the built-in function range(), which generates a sequence of numbers, from 0 up to -but not including- a specified number.

A range function has three parameters, which are: starting, ending and a step parameters. You can define each one of them as range(start, stop, step_size). This function does not store all the values in memory, it would be inefficient. So it remembers the start, stop, and step_size – then generates the next number, on the go.
Let’s take a look at how range() function can be used, with for loop.
for i in range(7):
print(i)
In the above example, the sequence starts from 0 and ends at 6 because the ending parameter is 7 and is not included. The default step is 1, if not provided. Therefore, the for execution jumps 1 step, for each new iteration, and will result in printing out all the numbers from 0 through 6 – included.
As you may have noticed, the range function starts from 0, by default. But, you can start the range at another number, by specifying the start parameter. This will result in printing out numbers from 2 through 6, included.
for i in range(2, 7):
print(i)
You can even generate a range of negative numbers, as well. This example will result in printing out numbers from -5 through -1, included – since the ending parameter is never included.
for i in range(-5, 0):
print(i)
Finally but not least, the range increments by 1 – by default. But, you can specify a different increment, by specifying a “step size” parameter. In the below example, the sequence starts from 2 and ends at 6, because the ending parameter is 7 – and the step is 2. Therefore, the for execution jumps 2 steps, after each iteration, and will result in printing out the numbers 2, 4 and 6.
for i in range(2, 7, 2):
print(i)
Access Index
Wow, that is a lot to take in. Right? Fortunately for you, this is the last part of this tutorial. You have seen how to iterate through strings, lists or any other iterable objects. But, you still don’t have any idea: How to get their respective index?
You can use the range() function in for loops, to iterate through a sequence of numbers. Plus, it can be combined with the len() function, to iterate through a sequence using indexing, as follows. This way, you can get the exact position of the current item, in the list.
colours = ['red', 'green', 'blue']
for index in range(len(colours)):
print(index, colours[index])
0 red
1 green
2 blue
That is Great! However, in most such cases, it is convenient to use the enumerate() function, which results in the same output, but simplify the way you write your Python code. Indeed, the enumerate() function will provide a tuple, that you can then unpack – to get both the index, and the value of the current item. Here is an example, with the same result.
colours = ['red', 'green', 'blue']
for index, value in enumerate(colours):
print(index, value)
Conclusion
In this tutorial, you have learned about a new structure, in Python: Iterations, also known as Loops. This is one of the statements which control the flow of execution in your program, and execute a block of code, repeatedly. All of these concepts are crucial to develop more advanced Python code, and reduce its complexity.
In the next tutorial, you will discover another loop statement: the while structure! This structure also facilitates iteration and repeats a statement, or block of statements, till some condition is met. So, Do not waste any minute of it, and join us in the next episode! If you liked this video, please, do not forget to give a thumb up, and subscribe our channel!
– Digital Academy™ 🎓
📖 Complete Python Development Course for Beginners
#Python #Tutorial #Beginners #For #Loops
***
♡ Thanks for watching and supporting ♡
Please Subscribe. Hit the notification bell.
Like, Comment and Share.
***
♡ FOLLOW US ♡
✧ http://digital.academy.free.fr/
✧ https://twitter.com/DigitalAcademyy
✧ https://www.facebook.com/digitalacademyfr
✧ https://www.instagram.com/digital_academy_fr
✧ https://www.youtube.com/c/DigitalAcademyOnline
***
♡ SUPPORT US ♡
✧ http://digital.academy.free.fr/join
✧ http://digital.academy.free.fr/donate
✧ http://digital.academy.free.fr/subscribe
✧ https://www.patreon.com/digital_academy
✧ https://www.buymeacoffee.com/digital_academy