Hello everyone, and Welcome back to Digital Academy – the Complete Python Development Tutorial for Beginners.
In the previous tutorial, you may have discovered in-depth use about Dictionary, in Python. This kind of Data Types is used, when you want to store a pair of “key:value”, and then access a value in a very efficient way – thanks to its key.
In this brand new video, you will now discover another Data Type: the List! Be sure to stick around, and watch until the end of this video, if you want to discover how you can: create a list, add and remove a few items, iterate through a list, seeking for and accessing a specific item – plus various built-in methods, that you can perform on lists.
Please, Do not forget to smash that Like button, for the YouTube algorithm – since it does really help supporting us, and providing new FREE content, once a week! You may also want to follow Digital Academy, on our other social platforms: Facebook, Instagram, Twitter and Patreon. This way, we could: stay in touch, grow this community, then share and help each other, throughout this exciting journey, OUR journey! Are you interested to be part of this community? All the Links are in the description below! Now, Let’s play this video …
Definition
Python offers a range of compound Data Types, often referred to as sequences. List is one of the most frequently used Data Types. In fact, List in Python is a collection of items, which is: ordered, indexed, allows duplicate members, and is mutable – so it can be changed, once it has been created. Consequently, it is often use when you want to store an item, and then access it in a very particular order.

The important properties of Python lists, are as follows:
- Lists are ordered – Lists remember the order of items inserted.
- Accessed by index – Items in a list can be accessed, using an index.
- Lists can contain any sort of object – It can be numbers, strings, tuples – and even other (nested) lists.
- Lists are dynamic – You can change a list in-place, add new items, and even delete or update existing items.
Creating a List
Great! Now that you have understood what a list is, let’s move forward and discover how to create a list in Python – but also what are all of the operations you can perform on it, in order to: add, remove, or access an item, or even search for a specific item inside of this list. So, be sure to stick around – and watch the entire video!
There are several ways to create a new list. In Python programming language, the simplest way to create a list is: to enclose all the items, also known as elements, inside square brackets [] – and separated by commas. It can have any number of items inside, and they may even be of different Data Types – integer, float, string, boolean, or even another nested list, which contains other items of different data types, and so forth.

Let’s have a closer look at its syntax, so you can declare your own list. Here is an example, in which you want to store a list of strings. First, Let’s declare an empty list – with empty brackets []. Eventually, you will add all of the strings, so that you can store and then access its values, later on.
# Empty list
my_list = []
# List of integers
my_list = [1, 2, 3]
# List of strings
my_list = ['red', 'green', 'blue']
# List with mixed data types
my_list = [1, "Hello", 3.4, True, [1, 2, 3]]
A list containing zero items is called an empty list, and you can create one with empty brackets []. But, you can also use the list() constructor to declare a new list in Python, or even convert other data types – like string or tuples, into a list.
# Convert string -> list
my_list = list('abc')
# my_list = ['a', 'b', 'c']
# Convert tuple -> list
my_list = list((1, 2, 3))
# my_list = [1, 2, 3]
Adding / Updating List
That’s great, but once you have created your list, you may also want to add other items, or update some values.
Adding or updating list’s items is very easy. Lists are mutable, which means their elements can be changed. In fact, you can add one item into a list, using the method append() – or add several items at once, using method extend().
To add new values to a list, use the method append(). This method adds items, only to the end of the list.
my_list = ['red', 'green', 'blue']
my_list.append('yellow')
# my_list = ['red', 'green', 'blue', 'yellow']
Furthermore, you can insert one item at a desired location, by using the method insert(). If you want to insert an item at a specific position in a list, you will use the insert() method. Note that: All of the values in the list after the inserted value will be moved down one index.
my_list = ['red', 'green', 'blue']
my_list.insert(1, 'yellow')
# my_list = ['red', 'yellow', 'green', 'blue']
Updating an entry to an existing list is simply a matter of assigning a new value, with the assignment operator (=). Thus, you can replace an existing element with its new value, by assigning the new value to the desired index. Note that: List indexing in Python is zero-based – as it is with strings.
my_list = ['red', 'green', 'blue']
my_list[0] = 'yellow'
# my_list = ['yellow', 'green', 'blue']
my_list[-1] = 'orange'
# my_list = ['yellow', 'green', 'orange']
Accessing an Item
Of course, list’s elements must be accessible, somehow! If you do not get them by key, like you would with dictionary in Python, then how would you get them?

You can think of a list as a relationship between indexes, and values. This relationship is called a mapping, where each index maps to one of the values. Thus, individual items in a list can be accessed using an index, in square brackets [].
This is exactly analogous to accessing individual characters, in a string. The index must be an integer, or it would result in TypeError. And, List indexing in Python is zero-based, as it is with strings. So, the first element of a list, will always be at the index zero. The indexes for the values in a list are illustrated as follow. For instance: a list having 5 elements will have an index vary from 0 through 4. And you can access individual items in a list, using its index – in square brackets.
my_list = ['red', 'green', 'blue', 'yellow', 'black']
my_list[0] # red
my_list[2] # blue
Nonetheless, If you refer to an index that is not in this list, you will get an Exception. To avoid such exception, Python will raise an “IndexError: list index out of range” error, if you use an index that exceeds the number of items in your list.
my_list = ['red', 'green', 'blue', 'yellow', 'black']
my_list[5] # IndexError: list index out of range
Negative List Indexing

Virtually everything about string indexing works similarly for lists. Thus, you can access a list by negative indexing, as well. For example, a negative list index counts from the end of the list. So, the index of -1 refers to the last item, -2 to the second last item, and so on. The negative indexes for the items in a list are illustrated as below.
my_list = ['red', 'green', 'blue', 'yellow', 'black']
my_list[-1] # black
my_list[-2] # yellow
Slicing a List

A segment of a list is called a slice. You can access or extract a range of items in a list, by using the slicing operator (:).

The slice operator my_list [ start : stop ] returns the part of the list from the “start-th” item to the “stop-th” item, which will include the first item but exclude the last one. Please note that a slice of a list, is also a list – and you may want to specify a step size parameter.

But, if you do not specify the start parameter, it will start from the beginning of this list! On the other hand, if you did not specify the end parameter, it will take all the items until it reaches the end of the list.
my_list = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i']
my_list[0:2] # ['a', 'b']
my_list[2:7] # ['c', 'd', 'e', 'f', 'g']
my_list[3:-1] # ['d', 'e', 'f', 'g', 'h']
my_list[2:] # ['c', 'd', 'e', 'f', 'g', 'h', 'i']
my_list[:5] # ['a', 'b', 'c', 'd', 'e']
Removing an Item
There are several ways to remove items from a list: either by its index, its value, or the last item in your list, and even remove all of them – at once.
Remove an Item by Index
If you know the index of the item you want, you can use the pop() method to remove an item, at a given index. It will: modify the list and returns the removed item. If there is no index specified, pop() removes and returns the last item in the list. This may help you implement lists as stacks – meaning first in, last out data structure.
my_list = ['red', 'green', 'blue']
item = my_list.pop(1)
# my_list = ['red', 'blue']
# item = green
If you do not need the removed value, you can delete one or more items from a list, using this specific keyword del.
my_list = ['red', 'green', 'blue']
del my_list[1]
# my_list = ['red', 'blue']
To remove more than one items, use the del keyword with a slice index.
my_list = ['red', 'green', 'blue', 'yellow', 'black']
del my_list[1:4]
# my_list = ['red', 'black']
Remove an Item by Value
If you are not sure where the item is in the list, you can use the remove() method to delete the given item, by value.
my_list = ['red', 'green', 'blue']
my_list.remove('red')
# my_list = ['green', 'blue']
But, keep in mind that if more than one instance of the given item is present, this method removes only the 1st instance
my_list = ['red', 'green', 'blue', 'red']
my_list.remove('red')
# my_list = ['green', 'blue', 'red']
Remove all Items
You can also use the clear() method, to empty a list. Use clear() method to remove all items from the list, at once. And the del keyword to delete the list itself, entirely.
my_list = ['red', 'green', 'blue']
my_list.clear()
# my_list = []
Checking existence
To determine whether a value is or is not in a list, you can use “in” and “not in” operators, combined with if statement. If you need help using this kind of statement, please check out the suggested videos: on operators, and conditionals.
# Checking for presence
my_list = ['red', 'green', 'blue']
if 'red' in my_list:
print('red is in my_list')
# Checking for absence
my_list = ['red', 'green', 'blue']
if 'yellow' not in my_list:
print('yellow is not in my_list')
Iterating through a List
Lists are a very useful and a widely used data structure, in Python. As a developer, you will often be in situations where you will need to iterate through a list, while you perform some actions on its items. So, the most common way to iterate through a list, is with a for loop.
my_list = ['red', 'green', 'blue']
for item in my_list:
print(item)
# OUTPUT
# red
# green
# blue
This works well if you only need to read the items of the list. But, if you want to update them, you need the indexes. A common way to do that, is to combine the range() and len() functions altogether. For instance, this loop iterates through the list and double each item. If you do not know how the range() function works, please check out a suggested video about: Python FOR Loops.
my_list = [1, 2, 3, 4]
for i in range(len(my_list)):
my_list[i] = my_list[i] * 2
# my_list = [2, 4, 6, 8]
List Length
Eventually, you may want to find how many items a list has. In that case, you do not have to iterate through the entire list and count each item, but use the function len() – which returns the number of items in a list.
my_list = ['red', 'green', 'blue']
print(len(my_list)) # OUTPUT: 3
Merging two Lists
You may sometimes want or need to merge two lists, so that you can have all of your data located in the same variable. Or, even update its values, with new content. In that case: use the built-in extend() method, to merge the values of one list, into another one. It takes a list as an argument, and appends all of the elements of this list, into the first list. Notice that: this method do not overwrite existing values, but add them at the end of your list.
As you can see in this example, merging the two lists will result in combining all of the items.
my_list = ['red', 'green', 'blue']
my_list.extend([1,2,3])
# my_list = ['red', 'green', 'blue', 1, 2, 3]
Alternatively, you can use the concatenation operator (+) or the augmented assignment operator (+=) to combine 2 lists. If you need help using operators, check out our Blog and the video about: Python Operators.
my_list = ['red', 'green', 'blue']
# Concatenation operator
my_list = my_list + [1,2,3]
# my_list = ['red', 'green', 'blue', 1, 2, 3]
# Augmented assignment operator
my_list += [1,2,3]
# my_list = ['red', 'green', 'blue', 1, 2, 3]
Nested Lists
A list can contain sublists as an item, which in turn can contain sublists themselves, and so on. This is known as nested list. You can use them to arrange data into hierarchical structures.
# Nested list
my_list = ["mouse", [8, 4, 6], ['a']]
Similarly, you can access individual items in a nested list, using multiple indexes. The first index determines which list to use, and the second indicates the value within that list. Indexes for the items in a nested list are illustrated as below.
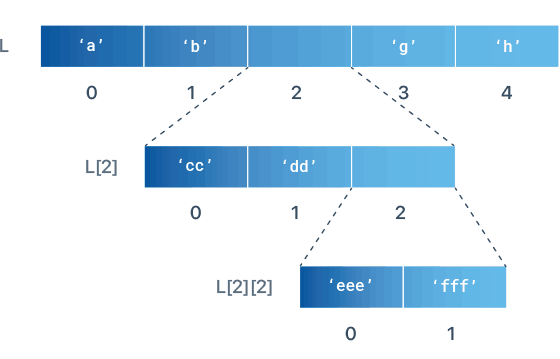
Keep in mind that: the deeper you are going to get an item, the more complex it will become, because of its syntax. So, it is sometimes more efficient to use another and more adapted Data Type.
my_list = ['a', 'b', ['cc', 'dd', ['eee', 'fff']], 'g', 'h']
print(my_list[2][2]) # OUTPUT: ['eee', 'fff']
print(my_list[2][2][0]) # OUTPUT: eee
Methods & Functions
Python has a set of other built-in methods, that you can call on list objects. Some of them have been used throughout this video, so you can add or insert new values, even get or remove a specific value. But, you may sometimes want to sort a list, duplicates list, count how many times an item is present inside your list, gets its min or max values, or even reverse it? Of course, all of that is possible in a very simple way, using these built-in methods. If you are interested to learn more about these methods, you can find more information on the Python website, and its documentation.
Method | Description |
---|---|
append() | Adds an item to the end of the list |
insert() | Inserts an item at a given position |
extend() | Extends the list by appending all the items from the iterable |
remove() | Removes first instance of the specified item |
pop() | Removes the item at the given position in the list |
clear() | Removes all items from the list |
copy() | Returns a shallow copy of the list |
count() | Returns the count of specified item in the list |
index() | Returns the index of first instance of the specified item |
reverse() | Reverses the items of the list in place |
sort() | Sorts the items of the list in place |
Python also has a set of built-in functions that you can use with list objects.
Method | Description |
---|---|
all() | Returns True if all list items are true |
any() | Returns True if any list item is true |
enumerate() | Takes a list and returns an enumerate object |
len() | Returns the number of items in the list |
list() | Converts an iterable (tuple, string, set etc.) to a list |
max() | Returns the largest item of the list |
min() | Returns the smallest item of the list |
sorted() | Returns a sorted list |
sum() | Sums items of the list |
Conclusion
In this tutorial, you have learned in-depth use about Lists, in Python. This kind of Data Types is used, when you want to store a few items of any Data Type, in a very specific order. Consequently, you should now be able to: create a list, add, update or remove items, and even search for a specific item. Thus, Lists should not be a secret anymore, and you can start using them in your program, straight away!
In the next tutorial, you will discover another Data Type, in details: Tuples! So, do not waste any minute of it, and join us in the next episode! If you liked this video, please: do not forget to give a thumb up, and subscribe our channel!
– Digital Academy™ 🎓
📖Complete Python Development Course for Beginners
#Python #Tutorial #Beginners #List
***
♡ Thanks for watching and supporting ♡
Please Subscribe. Hit the notification bell.
Like, Comment and Share.
***
♡ FOLLOW US ♡
✧ http://digital.academy.free.fr/
✧ https://twitter.com/DigitalAcademyy
✧ https://www.facebook.com/digitalacademyfr
✧ https://www.instagram.com/digital_academy_fr
✧ https://www.youtube.com/c/DigitalAcademyOnline
***
♡ SUPPORT US ♡
✧ http://digital.academy.free.fr/join
✧ http://digital.academy.free.fr/donate
✧ http://digital.academy.free.fr/subscribe
✧ https://www.patreon.com/digital_academy
✧ https://www.buymeacoffee.com/digital_academy