Hello everyone, and Welcome back to Digital Academy – the Complete Python Development Tutorial for Beginners.
In the previous tutorial, you may have discovered in-depth use about Tuples, in Python. This kind of Data Types is used, when you want to pack values and access them in a very efficient way, using the unpacking method – but also prevent its modification.
In this brand new video, you will now discover another Data Type: Sets! Be sure to stick around, and watch until the end of this video, if you want to discover various actions and methods that you can perform on Sets, in Python.

Please, Do not forget to smash that Like button, for the YouTube algorithm – since it does really help supporting us, and providing new FREE content, once a week! You may also want to follow Digital Academy, on our other social platforms: Facebook, Instagram, Twitter and Patreon. This way, we could: stay in touch, grow this community, then share and help each other, throughout this exciting journey, OUR journey! Are you interested to be part of this community? All the Links are in the description below! Now, Let’s play this video …
Definition
Python offers a range of compound Data Types, often referred to as sequences. Set is one of the most frequently used Data Types in Python – amongst List, Dictionary and Tuple. In fact, Set in Python is a collection of items, which is: not indexed, unordered, contains only unique elements, and is of mutable type – so it can be changed, grow and shrink, once it has been created. Moreover, they are commonly used for computing mathematical operations such as: union, intersection, difference, and symmetric difference operations.

The important properties of Python sets are as follows:
- Sets are unordered – Items stored in a set are not kept in any particular order.
- Set items are unique – Duplicate items are not allowed.
- Sets are unindexed – You cannot access set items by referring to an index.
- Sets are changeable (mutable) – They can be changed in place, can grow and shrink on demand.
Great! Now that you have understood what a Set is, let’s move forward and discover how to create a Set in Python – but also what are all of the operations you that can perform on it, in order to: add, remove, or access an item, even search for a specific item inside of this Set. So, be sure to stick around – and watch the entire video!
Create a Set
There are several ways to create a new Set. In Python programming language, the simplest way to create a Set is: to enclose all of the items, also known as elements, inside curly braces {} – and separated by commas. It can have any number of items inside, and they can even be of different Data Types – integer, float, string, boolean, or even another object, which contains other items of different data types, and so forth.
Although Set itself is mutable (changeable), it cannot contain mutable objects. Therefore, only immutable objects like Numbers, Strings, Tuples can be a Set item, but Lists and Dictionaries are mutable, so they cannot be.
Let’s have a closer look at its syntax, so you can declare your own Set. Here is an example, in which you want to store a list of strings. First, Let’s declare an empty Set – with empty curly braces {}. Eventually, you will add all of the strings, so that you can store and then access these values, later on.
# A set of strings
my_set = {'red', 'green', 'blue'}
# A set of mixed datatypes
my_set = {1, 'abc', 1.23, (3+4j), True}
But, Sets DO NOT allow duplicate members. Consequently, they are automatically removed – during its creation.
my_set = {'red', 'green', 'blue', 'red'}
# my_set = {'blue', 'green', 'red'}
A set is mutable (changeable), but it cannot contain mutable objects. Therefore, immutable objects like Numbers, Strings, Tuples can be a set item, but Lists and Dictionaries are mutable, so they cannot be.
my_set = {1, 'abc', ('a', 'b'), True}
my_set = {[1, 2], {'a':1, 'b':2}}
# Triggers TypeError: unhashable type: 'list'
Set constructor
As you have seen, a Set containing zero items is called an empty Set, and you can create one with empty curly braces {}. But, you can also create a Set using a type constructor called set(), to declare a new Set in Python. Or even convert other data types into a Set, like String, Tuples, or any object of immutable type.
# Set of items in an iterable
my_set = set('abc')
# my_set = {'a', 'b', 'c'}
# Set of successive integers
my_set = set(range(0, 4))
# my_set = {0, 1, 2, 3}
# Convert list/tuple into set
my_set = set([1, 2, 3])
my_set = set((1, 2, 3)
# my_set = {1, 2, 3}
That’s great, but once you have created your set, you may also want to add other items, or even update some values inside, right? Then, Let’s see how it all works, in the next section.
Add / Update Items to a Set
Adding or updating set’s items is very easy. Sets are mutable, which means their elements can be changed. In fact, you can add one single item into a Set, using the method add(). This method adds an item but not at the very end of the set – since sets are not indexed.
my_set = {'red', 'green', 'blue'}
my_set.add('yellow')
# my_set = {'blue', 'green', 'yellow', 'red'}
You can also add multiple items at once, using the method update().
my_set = {'red', 'green', 'blue'}
my_set.update(['yellow', 'orange'])
# my_set = {'blue', 'orange', 'green', 'yellow', 'red'}
Nonetheless, since a set is not indexed, you cannot update any value – once it has been added inside. Consequently, your only way to update an item in a Set would be to remove this value, then add another one. If you want to know how to remove an item from a set in Python, please follow along this video – and its next sections.
Access an Item
Of course, sets’s elements must be accessible, somehow! Unfortunately, Sets are not indexed, and unordered. If you do not get its values by keys nor indexes, like you would with a Dictionary or List in Python, then how would you get them? Well, the only way to access an item of a set in Python, is to iterate through every single item, using FOR and WHILE Loops. Eventually, you may also want to get a random item, using the method pop. Let’s move forward to the next sections, and discover how it works.
Remove Items from a Set
There are several ways to remove items from a set: either by its value, or a random item within your set – and even remove all of them, at once.
Remove an Item by Index
If you do not know the value of the item you want, you can use the pop() method to remove an item, located at a random position. It will: modify the set and returns the removed item.
my_set = {'red', 'green', 'blue'}
my_item = my_set.pop()
# my_set = {'green', 'red'}
# Removed item
print(my_item) # Blue
Remove an Item by Value
If you are not sure where the item is in the set and that you want to remove a specific and single value, but do not need to store it, you can use the remove() or discard() methods to delete this item by its value. But, keep in mind that: if more than one instance of the given item is present, this method removes only the 1st instance. Both methods work exactly the same. The only difference is that: If the specified item is not present in a set: the method remove() raises KeyError, whereas the method discard() does nothing.
# with remove() method
my_set = {'red', 'green', 'blue'}
my_set.remove('red')
# my_set = {'green', 'blue'}
# with discard() method
my_set = {'red', 'green', 'blue'}
my_set.discard('red')
# my_set = {'green', 'blue'}
Remove all Items
You can also use the clear() method, to permanently and fully remove all items from the set, at once. And the specific del keyword, to delete the set itself, entirely.
my_set = {'red', 'green', 'blue'}
my_set.clear()
# my_set = set()
Check if Item Exists in a Set
To determine whether a value is or is not in a Set, you can use “in” and “not in” operators, combined with if statement. If you need help using these kind of statements, please check out the suggested videos: on operators and conditionals.
# Check for presence
my_set = {'red', 'green', 'blue'}
if 'red' in my_set:
print('red is in my_set')
# Check for absence
my_set = {'red', 'green', 'blue'}
if 'yellow' not in my_set:
print('yellow is not in my_set')
Iterate through a Set
Sets are a very useful and a widely used data structure, in Python. As a developer, you will often be in situations where you will need to iterate through a set, while you perform some actions on its items. Therefore, the most common way to iterate through a Set, is using FOR Loop iterations.
my_set = {'red', 'green', 'blue'}
for my_item in my_set:
print(my_item)
# OUTPUT: red green blue
This works well if you only need to read the items of the Set. But, if you want to know at which position an item is, you will need the indexes. A common way to do that is to convert your set into a list, combine the functions range() and len() altogether, or use the enumerate() function. If you do not know how the enumerate() function works, please check out a suggested video about: Python FOR Loops.
Find Set Size
Eventually, you may want to find how many items a Set has. In that case, you do not have to iterate through the entire Set and count each item, but use the function len() – which returns the number of items in a Set.
my_set = {'red', 'green', 'blue'}
print(len(my_set)) # OUTPUT: 3
Join Two Sets
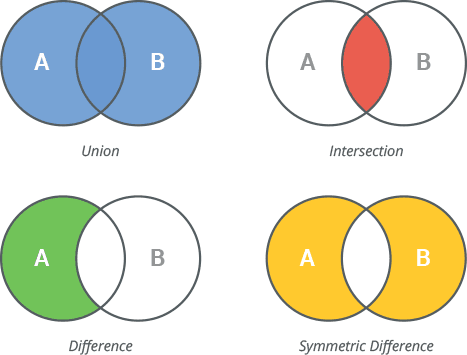
Sets are commonly used for computing mathematical operations such as intersection, union, difference, and symmetric difference. Consequently, there are several ways to join two or more sets, in Python.
Set Union
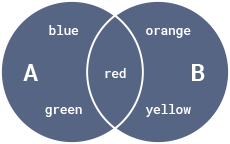
Union of the sets A and B is the set of all items in either A or B
You can use the method union(), that returns a new set containing all items from both sets, or the method update(), that inserts all the items from one set into another set. You can perform union on 2 or more sets using union() or “|” operator.
A = {'red', 'green', 'blue'}
B = {'yellow', 'red', 'orange'}
# by operator
my_new_set = (A | B)
# my_new_set = {'blue', 'green', 'yellow', 'orange', 'red'}
# by method
A.update(B)
my_new_set = A.union(B)
# my_new_set = {'blue', 'green', 'yellow', 'orange', 'red'}
There are other methods that joins two sets and keeps ONLY the duplicates or, on the opposite, NEVER keeps the duplicates…
Set Intersection

Intersection of the sets A and B is the set of items common to both A and B.
You can perform intersection on two or more sets using the method intersection() or “&” operator.
A = {'red', 'green', 'blue'}
B = {'yellow', 'red', 'orange'}
# by operator
my_new_set = (A & B)
# my_new_set = {'red'}
# by method
my_new_set = A.intersection(B)
# my_new_set = {'red'}
Set Difference
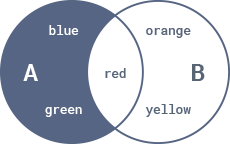
Set Difference of A and B is the set of all items that are in A but not in B.
You can compute the difference between two or more sets using the method difference() or “–” operator.
A = {'red', 'green', 'blue'}
B = {'yellow', 'red', 'orange'}
# by operator
my_new_set = (A - B)
# my_new_set = {'blue', 'green'}
# by method
my_new_set = A.difference(B)
# my_new_set = {'blue', 'green'}
Set Symmetric Difference

Symmetric difference of sets A and B is the set of all elements in either A or B, but not both.
You can compute symmetric difference between two or more sets using symmetric_difference() method or “^” (XOR) operator.
A = {'red', 'green', 'blue'}
B = {'yellow', 'red', 'orange'}
# by operator
my_new_set = (A ^ B)
# my_new_set = {'orange', 'blue', 'green', 'yellow'}
# by method
my_new_set = A.symmetric_difference(B)
# my_new_set = {'orange', 'blue', 'green', 'yellow'}
Built-in Functions with Set
Python has a set of other built-in functions and methods, that you can call on Sets objects. Some of them have been used throughout this video, so you can add or insert new values, even get or remove a specific value. But, you may sometimes want to sort a Set, count how many times an item is present inside your Set, gets its min or max values, or even determine whether a set is a subset of another set? Of course, all of that is possible in a very simple way, using these built-in methods. If you are interested to learn more about these methods, you can find more information on the Python website, and its documentation.
Method | Description |
union() | Return a new set containing the union of two or more sets |
update() | Modify this set with the union of this set and other sets |
intersection() | Returns a new set which is the intersection of two or more sets |
intersection_update() | Removes the items from this set that are not present in other sets |
difference() | Returns a new set containing the difference between two or more sets |
difference_update() | Removes the items from this set that are also included in another set |
symmetric_difference() | Returns a new set with the symmetric differences of two or more sets |
symmetric_difference_update() | Modify this set with the symmetric difference of this set and other set |
isdisjoint() | Determines whether or not two sets have any elements in common |
issubset() | Determines whether one set is a subset of the other |
issuperset() | Determines whether one set is a superset of the other |
Method | Description |
---|---|
all() | Returns True if all items in a set are true |
any() | Returns True if any item in a set is true |
enumerate() | Takes a set and returns an enumerate object |
len() | Returns the number of items in the set |
max() | Returns the largest item of the set |
min() | Returns the smallest item of the set |
sorted() | Returns a sorted set |
sum() | Sums items of the set |
Conclusion
In this tutorial, you have learned in-depth use about Sets, in Python. This kind of Data Types is used, when you want to compute mathematical operations, or do not store any duplicate members. Consequently, you should now be able to: create a Set, add or remove a few items, or even search for a specific item. Thus, Sets should not be a secret anymore, and you can start using them in your Python program, straight away!
In the next tutorial, you will finally discover Functions, so that you can arrange and reuse your code, in Python! So, do not waste any minute of it, and join us in the next episode! If you liked this video, please: do not forget to give a thumb up, and subscribe our channel!
– Digital Academy™ 🎓
📖 Complete Python Development Course for Beginners
#Python #Tutorial #Beginners #Set
***
♡ Thanks for watching and supporting ♡
Please Subscribe. Hit the notification bell.
Like, Comment and Share.
***
♡ FOLLOW US ♡
✧ http://digital.academy.free.fr/
✧ https://twitter.com/DigitalAcademyy
✧ https://www.facebook.com/digitalacademyfr
✧ https://www.instagram.com/digital_academy_fr
✧ https://www.youtube.com/c/DigitalAcademyOnline
***
♡ SUPPORT US ♡
✧ http://digital.academy.free.fr/join
✧ http://digital.academy.free.fr/donate
✧ http://digital.academy.free.fr/subscribe
✧ https://www.patreon.com/digital_academy
✧ https://www.buymeacoffee.com/digital_academy