Hello everyone, and Welcome back to Digital Academy – the Complete Python Development Tutorial for Beginners.
In the previous tutorials, you may have discovered Variables and Operators, or even Python Iterations with FOR and WHILE Loops, Conditionals IF Statements, or in-depth use of multiple Data Types (List, Dictionary, Tuple and Set).
In this brand new video, you will now discover a new way to write your own program, using: Functions! Functions are the first step to code reuse. Functions in Python allow you to define a block of code, that can then be used repeatedly, throughout your program. So be sure to stick around and watch until the end of this video if you want to discover more.
Please, Do not forget to smash that Like button, for the YouTube algorithm – since it does really help supporting us, and providing new FREE content, once a week! You may also want to follow Digital Academy, on our other social platforms: Facebook, Instagram, Twitter and Patreon. This way, we could: stay in touch, grow this community, then share and help each other, throughout this exciting journey, OUR journey! Are you interested to be part of this community? All the Links are in the description below! Now, Let’s play this video …
Definition – What is a Function in Python?
In Python, a function is a group of related statements, that performs a specific task. Functions help break your program into pieces of smaller and modular chunks. As your program grows, larger and larger, functions make it more organized and manageable. Furthermore, it avoids repetition, makes the code reusable, and allows you to work collaboratively.

That’s True! Functions are the first step to code reuse. They allow you to define a reusable block of code, that can be used, repeatedly, in your program. Actually, Python provides several built-in functions, such as: print(), len() or type(). But, you can also define your own functions to use, within your programs.
Now, Let’s have a closer look at its Syntax, with an example.
Syntax
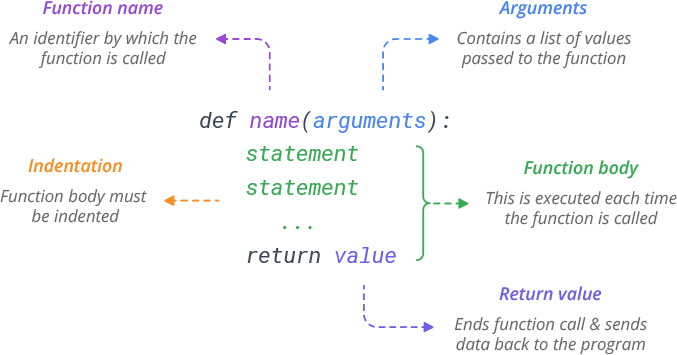
The basic syntax for a Python function definition is as follows:
- Keyword def, that marks the start of the function header.
- Function name, to uniquely identify the function throughout your program.
- Optional parameters (arguments), through which you pass values to a function.
- Colon (:), to mark the end of the function header.
- Optional documentation string (docstring) to describe what the function does.
- One or more valid Python statements in the function body, with the same indentation level – usually 4 spaces.
- Optional return statement, to return a value from the function.
Example of a function in Python
def hello(name):
"""
This function greets to
the person passed in as
a parameter
"""
print(f"Hello, {name}. Keep watching!")
Create a Function
To define a Python function, use the keyword def, followed by your function name with parenthesis and one colon. Here is the simplest possible function, that prints out: ‘Hello, World!’
def hello():
print('Hello, World!')
Pretty simple, right? Then, Let’s move forward to the next section, and discover how to call this function.
Call a Function
The def statement only creates a function, but does not call it, afterwards. You must call (run) the function explicitly, by adding parentheses after the function’s name. Consequently, once you have defined your function, you can call it from another function, and anywhere in your program – or even from the Python interpreter.
def hello():
print('Hello, World!')
hello() # OUTPUT: Hello, World!
Awesome, you can now define your own block of code in Python, also known as function. You can call this function from anywhere in your program. But, what is the point, if this bock of code cannot be customised, with user-defined values?! Well, Let’s see how argument works, and how to pass these arguments to your function.
Pass Arguments to a Function
You can send information to a function, by passing values – known as arguments. Arguments are declared after the function name, in parentheses. When you call a function with arguments, the values of those arguments are copied into their corresponding parameters, inside the function.
# Pass single argument to a function
def hello(name):
print('Hello,', name)
hello('Jérémy') # OUTPUT: Hello, Jérémy
hello('Digital Academy') # OUTPUT: Hello, Digital Academy
Obviously, you can send as many arguments as you like, separated by commas “,”.
# Pass two arguments
def func(name, job):
print(name, 'is a', job)
func('Jérémy', 'YouTuber') # OUTPUT: Jérémy is a YouTuber
Types of Arguments
Python handles function arguments in a very flexible manner, compared to other languages. It supports multiple types of arguments in the function definition. Here is the complete list:
- Positional Arguments
- Keyword Arguments
- Default Arguments
- Variable Length Positional Arguments (*args)
- Variable Length Keyword Arguments (**kwargs)
Positional Arguments
The most common are positional arguments, whose values are copied to their corresponding parameters – in order.
def func(name, job):
print(name, 'is a', job)
func('Jérémy', 'YouTuber') # OUTPUT: Jérémy is a YouTuber
The only downside of positional arguments is that you need to pass arguments in the order in which they are defined.
def func(name, job):
print(name, 'is a', job)
func('YouTuber', 'Jérémy') # OUTPUT: YouTuber is a Jérémy
Keyword Arguments
To avoid positional argument confusion, you can pass arguments using the names of their corresponding parameters.
In this case, the order of the arguments no longer matters, because arguments are matched by name – not by position.
# Keyword arguments can be put in any order
def func(name, job):
print(name, 'is a', job)
func(name='Jérémy', job='developer')
# OUTPUT: Jérémy is a developer
func(job='YouTuber', name='Digital Academy')
# OUTPUT: Digital Academy is a YouTuber
Please note that: It is also possible to combine positional and keyword arguments, in a single call. If you do so, specify the positional arguments before keyword arguments.
Default Arguments
You can specify default values for arguments when defining a function. The default value is used if the function is called without a corresponding argument. In short, defaults allow you to make selected arguments optional.
# Set default value 'developer' to a 'job' parameter
def func(name, job='developer'):
print(name, 'is a', job)
func('Jérémy', 'YouTuber')
# OUTPUT: Jérémy is a YouTuber
func('Jérémy')
# OUTPUT: Jérémy is a developer
Variable Length Arguments
Variable length arguments are very useful, when you want to create functions that take unlimited number of arguments. Unlimited, in the sense that: you don’t know beforehand how many arguments can be passed to a function by the user.
*args
When you prefix a parameter with an asterisk * , it collects all the unmatched positional arguments, into a tuple. Because it is a normal tuple object, you can perform any operation that a tuple supports, like indexing, iteration etc.
Following function prints all the arguments passed to the function as a tuple.
def print_arguments(*args):
print(args)
print_arguments(1, 54, 60, 8, 98, 12)
# OUTPUT: (1, 54, 60, 8, 98, 12)
Note: You do not need to call this keyword parameter args, but it is standard practice.
**kwargs
The ** syntax is similar, but it only works for keyword arguments. It collects them into a new dictionary, where the argument names are the keys, and their values are the corresponding dictionary values.
def print_arguments(**kwargs):
print(kwargs)
print_arguments(name='Jérémy', age=27, job='developer')
# OUTPUT: {'name': 'Jérémy', 'age': 27, 'job': 'developer'}
Pretty awesome, right? You can now define your own function, and use all kind of arguments: positional and keyword arguments, with or without default values – and even variable length arguments, using *args and **kwargs keywords.
That’s Great, Jérémy! But, again, what’s the point if I defined a function, but I cannot get back its result? Hopefully, Jérémy from Digital Academy is here to help you out. Let’s move forward, and discover the return statement.
Return Values

The return statement in Python, is used to exit a function – and go back to the place from where it was called. This statement can contain an expression that gets evaluated, and the value is returned. If there is no expression in the statement or the return statement itself is not present inside a function, then the function will return the None object. Here, None is returned since hello() directly prints the name passed as argument – and no return statement is used.
def hello(name):
print(f'Hello, {name}!')
Return Single Value
To return a value from a function, simply use a return statement. Once a return statement is executed, nothing else in the function body will be executed. Remember: Python function always returns a value. Therefore, If you do not include any return statement, it automatically returns None. And, ALL variables declared in this function, will not be accessible outside the function (variable scope).
# Return sum of two values
def sum(a, b):
return a + b
x = sum(3, 4) # x = 7
Return Multiple Values
Python has the ability to return multiple values, something missing from many other languages. Fortunately for you, you can simply do this, by separating return values with a comma.
# Return addition and subtraction in a tuple
def func(a, b):
return a+b, a-b
result = func(3, 2) # result = (5, 1)
When you return multiple values, Python actually packs them into a single tuple, and returns it. Consequently, you can then use multiple assignment to unpack the parts of the returned tuple, into multiple variables. (See Tuple)
# Unpack returned tuple
def func(a, b):
return a+b, a-b
add, sub = func(3, 2)
print(add) # OUTPUT: 5
print(sub) # OUTPUT: 1
Docstring
Although optional, documentation is a good programming practice – especially if you want to get your dreamed job at Google, Apple, Facebook or anywhere in the World – and work collaboratively! Plus, unless you can remember what you had for dinner last week, always documenting your code will help you a lot when you will need to stay away for a while, then come back.
You can attach documentation to a function definition by including a string literal called docstring, just after the function header. It is briefly used to explain what a function does. Note: Docstrings are usually triple quoted, to allow for multi-line descriptions.
def hello():
"""This function prints
message on the screen"""
print('Hello, World!')
To print a function’s docstring, use the Python help() function – and pass the function’s name.
# Print docstring in rich format
help(hello)
# Help on function hello in module __main__:
# hello()
# This function prints
# message on the screen
You can also access the docstring through doc attribute of the function.
# Print docstring in a raw format
print(hello.__doc__)
# OUTPUT: This function prints message on the screen
Nested Functions
A Nested function is a function defined within another function. They are useful when performing complex task multiple times within another function, to avoid loops and code duplication outside.

def outer(a, b):
def inner(c, d):
return c + d
return inner(a, b)
result = outer(2, 4) # result = 6
Recursion
A recursive function, is a function that calls itself, and repeats its behaviour, until some condition is met, to return a result.
In this example, countdown() is a recursive function that calls itself, recursively, to countdown. If number is 0 or negative, it prints the word “Stop” then automatically exits this function. Otherwise, it prints number, and then calls itself agin, passing “number-1” as the new argument.
def countdown(num):
if num <= 0:
print('Stop')
else:
print(num)
countdown(num-1)
countdown(5)
# OUTPUT: 5
# OUTPUT: 4
# OUTPUT: 3
# OUTPUT: 2
# OUTPUT: 1
# OUTPUT: Stop
Conclusion
In this complete tutorial, you have learned Functions, in Python. Consequently, you should now be able to: define your own function, pass different kind of arguments to you function, and get its returned value. Thus, Functions should not be a secret anymore, and you can start using them in your Python program, straight away!
In the next tutorial, you will finally discover: Exceptions in Python, so that you can finally catch errors in your program without even interrupting its execution flow! So, do not waste any minute, and join us in the next episode! If you liked this video, Please: Do not forget to give a Thumb up, and Subscribe our channel!
– Digital Academy™ 🎓
📖 Complete Python Development Course for Beginners
#Python #Tutorial #Beginners #Function
***
♡ Thanks for watching and supporting ♡
Please Subscribe. Hit the notification bell.
Like, Comment and Share.
***
♡ FOLLOW US ♡
✧ http://digital.academy.free.fr/
✧ https://twitter.com/DigitalAcademyy
✧ https://www.facebook.com/digitalacademyfr
✧ https://www.instagram.com/digital_academy_fr
✧ https://www.youtube.com/c/DigitalAcademyOnline
***
♡ SUPPORT US ♡
✧ http://digital.academy.free.fr/join
✧ http://digital.academy.free.fr/donate
✧ http://digital.academy.free.fr/subscribe
✧ https://www.patreon.com/digital_academy
✧ https://www.buymeacoffee.com/digital_academy